Game Development Reference
In-Depth Information
Finding out if the Player Wants to Play Again
40. def playAgain():
41. # This function returns True if the player wants to
play again, otherwise it returns False.
42. print('Do you want to play again? (yes or no)')
43. return input().lower().startswith('y')
The
playAgain()
function is the same one we used in Hangman and Tic Tac Toe.
The long expression on line 43 will evaluate to either
True
or
False
. The return value
from the call to the
input()
function is a string that has its
lower()
method called on
it. The
lower()
method returns another string (the lowercase string) and that string has its
startswith()
method called on it, passing the argument
'y'
.
The Start of the Game
45. NUMDIGITS = 3
46. MAXGUESS = 10
47.
48. print('I am thinking of a %s-digit number. Try to guess
what it is.' % (NUMDIGITS))
49. print('Here are some clues:')
50. print('When I say: That means:')
51. print(' Pico One digit is correct but in the
wrong position.')
52. print(' Fermi One digit is correct and in the
right position.')
53. print(' Bagels No digit is correct.')
This is the actual start of the program. Instead of hard-coding three digits as the size of
the secret number, we will use the constant variable
NUMDIGITS
. And instead of hard-
coding a maximum of ten guesses that the player can make, we will use the constant
variable
MAXGUESS
. (This is because if we increase the number of digits the secret number
has, we also might want to give the player more guesses. We put the variable names in all
capitals to show they are meant to be constant.)
The
print()
function call will tell the player the rules of the game and what the Pico,
Fermi, and Bagels clues mean. Line 48's
print()
call has
% (NUMDIGITS)
added to
the end and
%s
inside the string. This is a technique know as string interpolation.
String Interpolation
String interpolation is another shortcut, like augmented assignment operators. Normally,
if you want to use the string values inside variables in another string, you have to use the
+
concatenation operator:
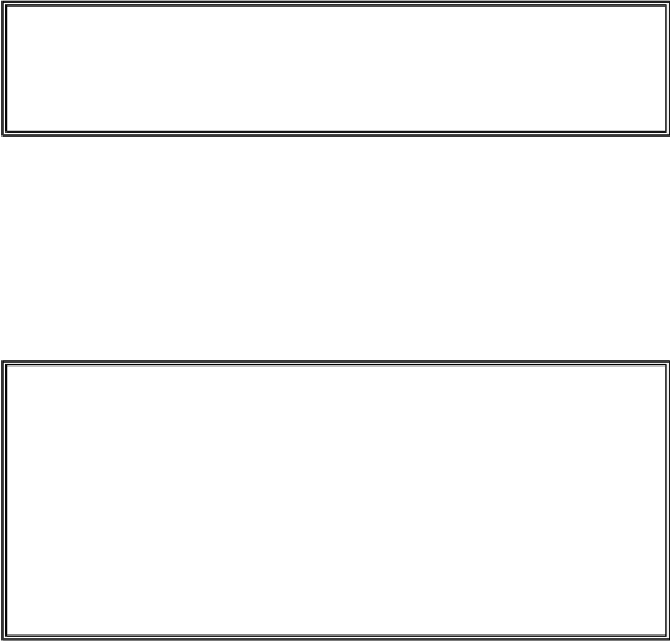