Game Development Reference
In-Depth Information
go to the fourth step.
Fourth, check if the center is free. If so, move there. If it isn't, then go to the fifth step.
Fifth, move on any of the side pieces (spaces 2, 4, 6, or 8). There are no more steps,
because if we have reached this step then the side spaces are the only spaces left.
The Computer Checks if it Can Win in One Move
103. # Here is our algorithm for our Tic Tac Toe AI:
104. # First, check if we can win in the next move
105. for i in range(1, 10):
106. copy = getBoardCopy(board)
107. if isSpaceFree(copy, i):
108. makeMove(copy, computerLetter, i)
109. if isWinner(copy, computerLetter):
110. return i
More than anything, if the computer can win in the next move, the computer should
immediately make that winning move. We will do this by trying each of the nine spaces on
the board with a
for
loop. The first line in the loop makes a copy of the
board
list. We
want to make a move on the copy of the board, and then see if that move results in the
computer winning. We don't want to modify the original Tic Tac Toe board, which is why
we make a call to
getBoardCopy()
. We check if the space we will move is free, and if
so, we move on that space and see if this results in winning. If it does, we return that
space's integer.
If moving on none of the spaces results in winning, then the loop will finally end and we
move on to line 112.
The Computer Checks if the Player Can Win in One Move
112. # Check if the player could win on his next move, and
block them.
113. for i in range(1, 10):
114. copy = getBoardCopy(board)
115. if isSpaceFree(copy, i):
116. makeMove(copy, playerLetter, i)
117. if isWinner(copy, playerLetter):
118. return i
At this point, we know we cannot win in one move. So we want to make sure the human
player cannot win in one more move. The code is very similar, except on the copy of the
board, we place the player's letter before calling the
isWinner()
function. If there is a
position the player can move that will let them win, the computer should move there to
block that move.
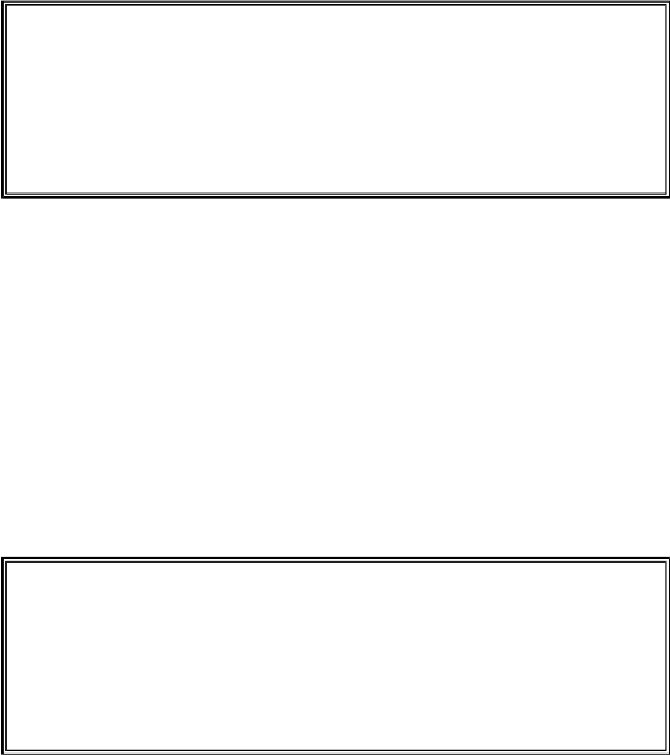