Java Reference
In-Depth Information
aPoint and bPoint are at the same location.
How It Works
You apply the three constructors that the
Point
class provides in the first few lines. You then manipulate
the
Point
objects you've instantiated.
You change a
Point
object to a new position with the
move()
method. Alternatively, you can use the
setLocation()
method to set the values of the
x
and
y
members. The
setLocation()
method be-
haves exactly the same as the
move()
method. It's included in the
Point
class for compatibility with the
setLocation()
method for a component. For the same reason, there's also a
getLocation()
method in
the
Point
class that returns a copy of the current
Point
object. As the example shows, you can translate
a
Point
object by specified distances in the
x
and
y
directions using the
translate()
method.
Lastly, you compare two
Point
objects using the
equals()
method. This compares the
x
and
y
coordin-
ates of the two
Point
objects and returns
true
if both are equal. The final output statement is executed
because the
Point
objects are equal.
Note that this is not the only class that represents points. You see other classes that define points in
Chapter 19 when I discuss how you draw in a window.
Rectangle Objects
As I mentioned earlier, the
java.awt.Rectangle
class defines four
public
data members, all of type
int
.
The position of a
Rectangle
object is defined by the members
x
and
y
, and its size is defined by
width
and
height
. As they are all
public
, you can retrieve or modify these directly, but your code may be a little more
readable if you use the methods provided to access them.
There are no less than seven constructors that you can use:
•
Rectangle()
creates a rectangle at (0,0) with zero width and height.
•
Rectangle(int x,int y,int width,int height)
creates a rectangle at (
x
,
y)
with the speci-
fied width and height.
•
Rectangle(int width, int height)
creates a rectangle at (0,0) with the specified width and
height.
•
Rectangle(Point p, Dimension d)
creates a rectangle at point
p
with the width and height
specified by
d.
A
java.awt.Dimension
object has two public fields,
width
and
height
.
•
Rectangle(Point p)
creates a rectangle at point
p
with zero width and height.
•
Rectangle(Dimension d)
creates a rectangle at (0,0) with the width and height specified by
d.
•
Rectangle(Rectangle r)
creates a rectangle with the same position and dimensions as the rect-
angle
r
.
You can retrieve or modify the position of a
Rectangle
object using the
getLocation()
method that
returns a
Point
object, and
setLocation()
, which comes in two versions, one of which requires
x
and
y
coordinates of the new position as arguments and the other of which requires a
Point
object. You can also
apply the
translate()
method to a
Rectangle
object in the same way as to a
Point
object.
To retrieve or modify the size of a
Rectangle
object, you use the methods
getSize()
, which returns a
Dimension
object, and
setSize()
, which requires either a
Dimension
object specifying the new size as an
argument or two arguments corresponding to the new width and height values as type
int
.
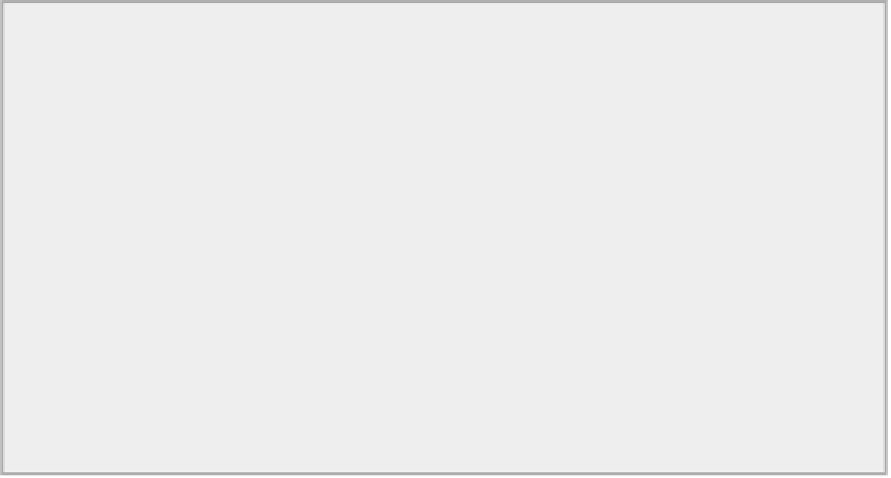