Java Reference
In-Depth Information
question
How It Works
The program produces 10 tokens in the output, which is the number of words in the text. The original
version in Chapter 4 treated a comma followed by a space as two separate tokens and produced an empty
token as a result. The pattern
"[^\\w]+"
matches one or more characters that are not word characters;
i.e., not uppercase or lowercase letters, or digits. This means the delimiters pattern includes one or more
spaces, periods, exclamations marks and question marks, and all the words in
text
are found.
Search and Replace Operations
You can implement a search and replace operation very easily using regular expressions. Whenever you call
the
find()
method for a
Matcher
object, you can call the
appendReplacement()
method to replace the
subsequence that was matched. You create a revised version of the original string in a new
StringBuffer
object that you supply to the method. The arguments to the
appendReplacement()
method are a reference
to the
StringBuffer
object that is to contain the new string, and the replacement string for the matched
text. You can see how this works by considering a specific example.
Suppose you define a string to be searched as:
String joke = "My dog hasn't got any nose.\n" +
"How does your dog smell then?\n" +
"My dog smells horrible.\n";
You now want to replace each occurrence of
"dog"
in the string by
"goat"
. You first need a regular ex-
pression to find
"dog"
:
String regEx = "dog";
You can compile this into a pattern and create a
Matcher
object for the string
joke
:
Pattern doggone = Pattern.compile(regEx);
Matcher m = doggone.matcher(joke);
You are going to assemble a new version of
joke
in a
StringBuffer
object that you can create like this:
StringBuffer newJoke = new StringBuffer();
This is an empty
StringBuffer
object ready to receive the revised text. You can now find and replace
instances of
"dog"
in
joke
by calling the
find()
method for
m
and calling
appendReplacement()
each
time it returns
true
:
while(m.find()) {
m.appendReplacement(newJoke, "goat");
}
Each call of
appendReplacement()
copies characters from
joke
to
newJoke
starting at the character
where the previous
find()
operation started and ending at the character preceding the first character
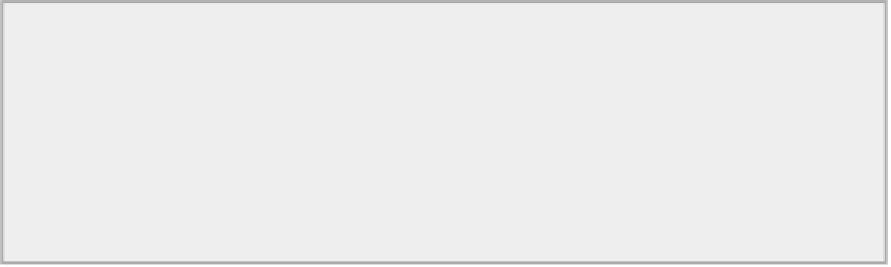