Java Reference
In-Depth Information
as changed by calling the
setChanged()
method. The current object and the argument passed to
the
notifyObservers()
method are passed to the
update()
method for each
Observer
object.
The
clearChanged()
method for the
Observable
is called to reset its status.
•
void notifyObservers()
: Calling this method is equivalent to calling the previous method with
a
null
argument.
•
int countObservers()
: Returns the number of
Observer
objects for the current object.
•
void setChanged()
: Sets the current object as changed. You must call this method before calling
the
notifyObservers()
method. Note that this method is
protected
.
•
boolean hasChanged()
: Returns
true
if the object has been set as changed and returns
false
otherwise.
•
void clearChanged()
: Resets the changed status of the current object to unchanged. Note that
this method is also
protected
. This method is called automatically after
notifyObservers()
is
called.
It's fairly easy to see how these methods are used to manage the relationship between an
Observable
object and its observers. To connect an observer to an
Observable
object, the
Observer
object must be re-
gistered with the
Observable
object by calling its
addObserver()
method. The
Observer
is then notified
when changes to the
Observable
object occur. An
Observable
object is responsible for adding
Observer
objects to its internal record through the
addObserver()
method. In practice, the
Observer
objects are typ-
ically created as objects that are dependent on the
Observable
object, and then they are added to the record,
so there's an implied ownership relationship.
This makes sense if you think about how the mechanism is often used in an application using the doc-
ument/view architecture. A document has permanence because it represents the data for an application. A
view is a transient presentation of some or all of the data in the document, so a document object should nat-
urally create and own its view objects. A view is responsible for managing the interface to the application's
user, but the update of the underlying data in the document object would be carried out by methods in the
document object, which would then notify other view objects that a change has occurred.
Of course, you're in no way limited to using the
Observable
class and the
Observer
interface in the way
in which I described here. You can use them in any context where you want changes that occur in one class
object to be communicated to others. We can exercise the process in a frightening example.
TRY IT OUT: Observing the Observable
We first define a class for an object that can exhibit change:
import java.util.Observable;
public class JekyllAndHyde extends Observable {
public void drinkPotion() {
name = "Mr.Hyde";
setChanged();
notifyObservers();
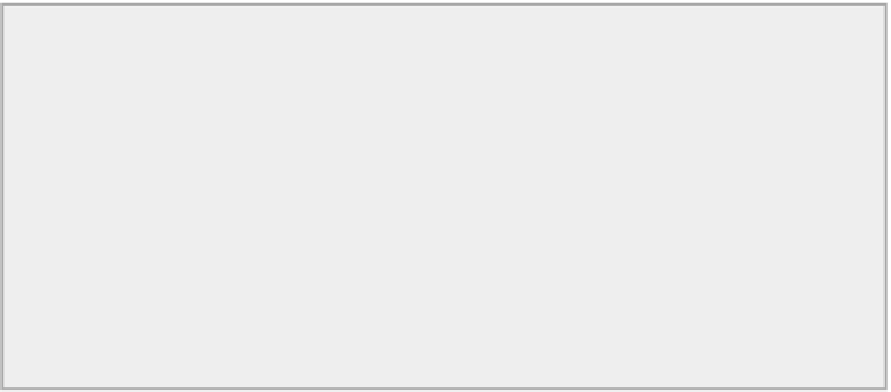
