Java Reference
In-Depth Information
However, you could use a
static
method to read a number from the keyboard, so let's add that, too:
import java.io.*;
class PhoneNumber implements Serializable {
// Read a phone number from the keyboard
public static PhoneNumber readNumber() {
String area = null;
// Stores the
area code
String localcode = null;
// Stores the
local code
try {
System.out.print("Enter area code: ");
area = keyboard.readLine().trim();
System.out.print("Enter local code: ");
localcode = keyboard.readLine().trim();
System.out.print("Enter the number: ");
localcode += " " + keyboard.readLine().trim();
} catch(IOException e) {
System.err.println("Error reading a phone number.");
e.printStackTrace();
System.exit(1);
}
return new PhoneNumber(area,localcode);
}
// Rest of the class as before...
private static BufferedReader keyboard = new BufferedReader(
new
InputStreamReader(System.in));
}
Directory "TryPhoneBook 1"
This is again very similar to the
readPerson()
method. You don't need a separate variable to store the
number that is entered. You just append the string that is read to
localcode
, with a space character in-
serted to make the output look nice. In practice, you certainly want to verify that the input is valid, but
you don't need this to show how a hash map works.
An entry in the phone book combines the name and the number and probably includes other things such
as the address. You can get by with the basics:
import java.io.Serializable;
class BookEntry implements Serializable {
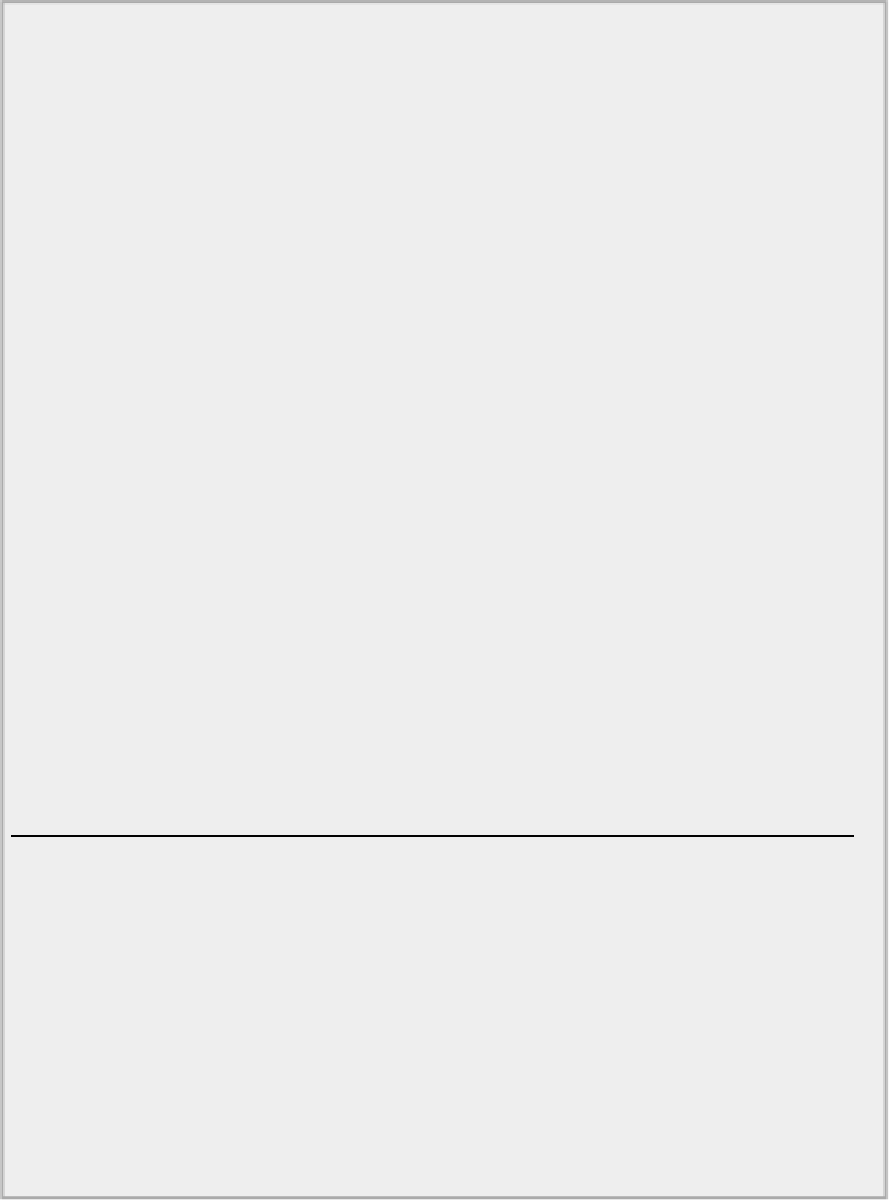


