Java Reference
In-Depth Information
The class is a lot simpler because the
LinkedList<>
class provides all the mechanics for operating a
linked list. Because the interface to the
PolyLine
class is the same as the previous version, the original
version of
main()
runs unchanged and produces exactly the same output.
How It Works
The only interesting bit is the change to the
PolyLine
class.
Point
objects are now stored in the linked
list implemented by the
LinkedList<>
object,
polyline
. You use the
add()
method to add points in
the constructors, and the
addPoint()
methods. Using a collection class makes the
PolyLine
class very
straightforward.
I changed the implementation of the second constructor in the
PolyLine
class to illustrate how you can
use the collection-based
for
loop with a two-dimensional array:
public PolyLine(double[][] coords) {
for(double[] xy : coords) {
addPoint(xy[0], xy[1]);
}
}
The
coords
parameter to the constructor is a two-dimensional array of elements of type
double
. This is
effectively a one-dimensional array of references to one-dimensional arrays that have two elements each,
corresponding to the
x
and
y
coordinate values for a point. Thus, you can use the collection-based
for
loop to iterate over the array of arrays. The loop variable is
xy
, which is of type
double[]
and has two
elements. Within the loop, you pass the elements of the array
xy
as arguments to the
addPoint()
method.
This method then creates a
Point
object and adds it to the
LinkedList<Point>
collection,
polyline
.
USING MAPS
As you saw at the beginning of this chapter, a
map
is a way of storing data that minimizes the need for
searching when you want to retrieve an object. Each object is associated with a key that is used to determine
where to store the reference to the object, and both the key and the object are stored in the map. Given a
key, you can always go more or less directly to the object that has been stored in the map based on the key.
It's important to understand a bit more about how the storage mechanism works for a map, and in particular
what the implications of using the default hashing process are. You explore the use of maps primarily in the
context of the
HashMap<K,V>
generic class type.
The Hashing Process
The implementation of a map in the Java collections framework that is provided by the
HashMap<K,V>
class
sets aside an array in which it stores key and object pairs of type
K
and
V
respectively. The index to this array
is produced from the key object by using the hashcode for the object to compute an offset into the array for
storing the key/object pair. By default, this uses the
hashCode()
method for the key object. This is inherited
in all classes from
Object
so this is the method that produces the basic hashcode unless the
hashCode()
method is redefined in the class for the key. The
HashMap<>
class does not assume that the basic hashcode is
adequate. To try to ensure that the hashcode that is used has the characteristics required for an efficient map,
the basic hashcode is further transformed within the
HashMap<>
object.
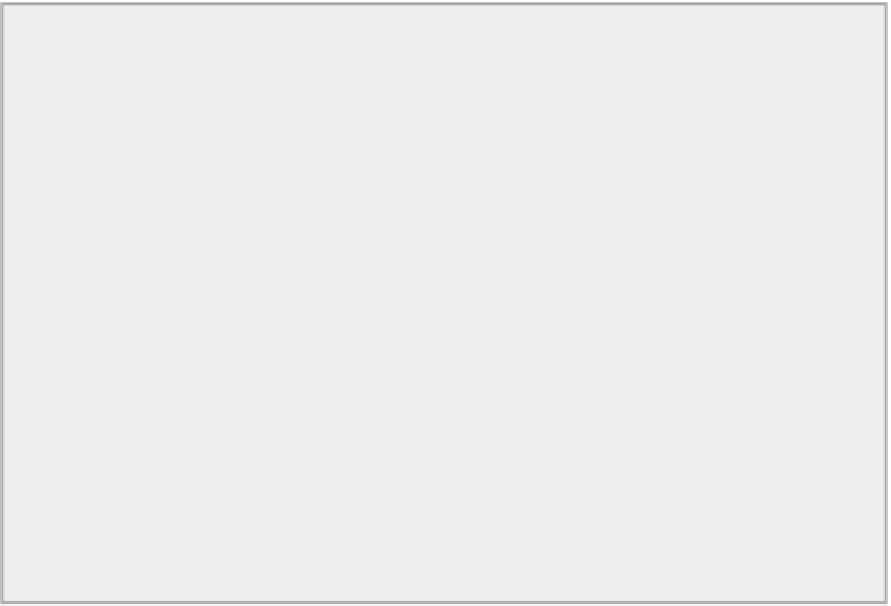