Java Reference
In-Depth Information
listAll(people.sort()); // List the sorted contents of the tree
The argument to the
listAll()
method is of type
BinaryTree<Person>
, so the compiler supplies
Per-
son
as the type argument to the method. This means that within the method, the loop iterates over an
array of
Person
references using a loop variable of type
Person
. The output demonstrates that the mix
of
Person
and
Manager
objects were added to the binary tree correctly and are displayed in the correct
sequence.
Generic Constructors
As you know, a constructor is a specialized kind of method and you can define class constructors with their
own independent parameters. You can define parameterized constructors for both ordinary classes and gen-
eric class types. Let's consider an example.
Suppose you want to add a constructor to the
BinaryTree<T>
type definition that accepts an argument
that is an array of items to be added to the binary tree. In this case, defining the constructor as a parameter-
ized method gives you the same flexibility that you have with the
add()
method. Here's how the constructor
definition looks:
public <E extends T> BinaryTree(E[] items) {
for(E item : items) {
add(item);
}
}
The constructor type parameter is
E
. You have defined this with an upper bound of
T
, so the argument to
the constructor can be an array of elements of the type specified by the type parameter
T
or any subclass of
T
. For example, if you define a binary tree of type
BinaryTree<Person>
, then you can pass an array to the
constructor with elements of type
Person
or any type that is a subclass of
Person
.
Let's try it.
TRY IT OUT: Using a Parameterized Constructor
The definition of
BinaryTree<>
is now as follows:
public class BinaryTree<T extends Comparable<? super T>> {
// No-arg constructor
public BinaryTree() {}
// Parameterized constructor
public <E extends T> BinaryTree(E[] items) {
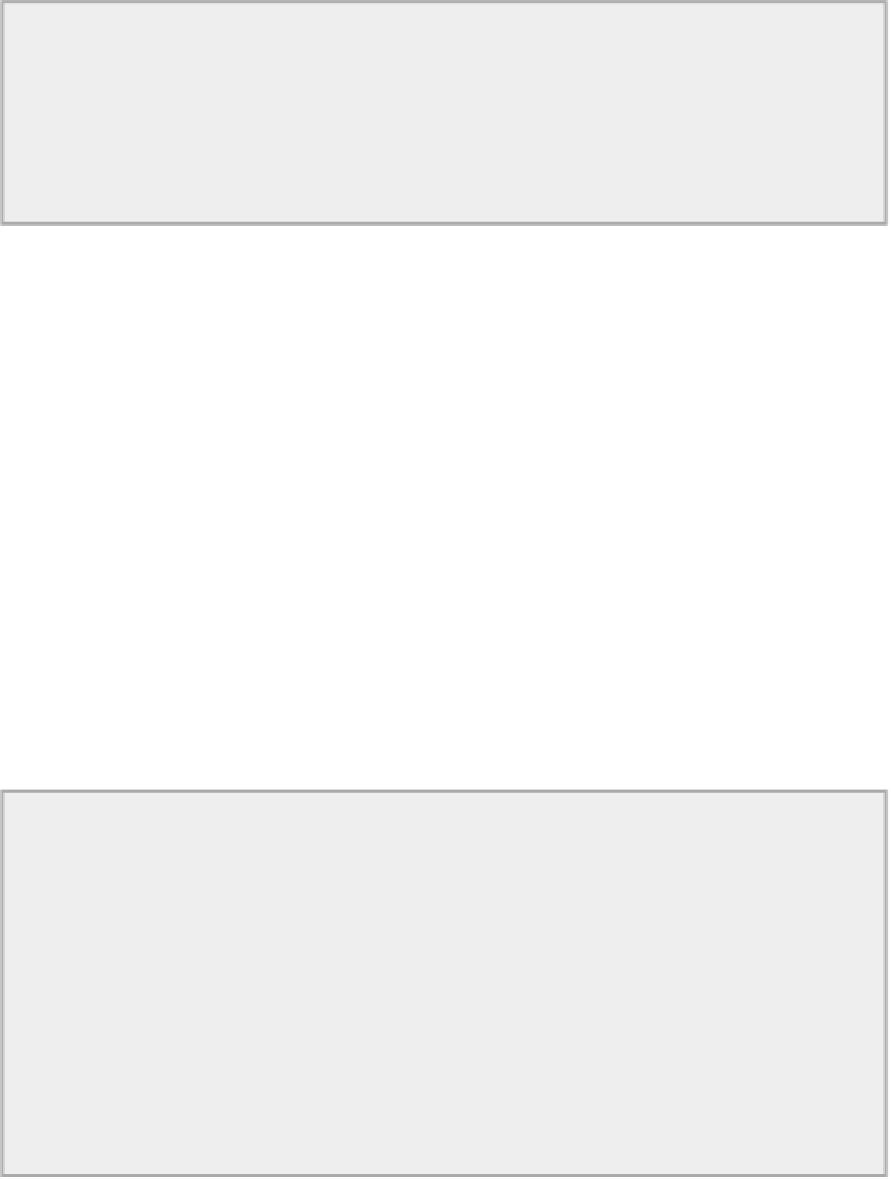
