Java Reference
In-Depth Information
you are transferring large amounts of data, this is potentially much faster than using explicit read and
write operations. How much faster depends on how efficiently your operating system handles memory-
mapped files and whether the way in which you access the data results in a large number of page faults.
Memory-mapped files have one risky aspect that you need to consider, and you will look at that in the
next section.
Locking a File
You need to take care that an external program does not modify a memory-mapped file that you are working
with, especially if the file could be truncated externally while you are accessing it. If you try to access a part
of the file through a
MappedByteBuffer
that has become inaccessible because a segment has been chopped
off the end of the file by another program, then the results are somewhat unpredictable. You may get a junk
value back that your program may not recognize as such, or an exception of some kind may be thrown. You
can acquire a
lock
on the file to try to prevent this sort of problem. A
sharedlock
allows several processes to
have concurrent read access to the file. An
exclusive lock
gives you exclusive access to the file; no one else
can access the file while you have the exclusive lock. A file lock simply ensures your right of access to the
file and might also inhibit the ability of others to change or possibly access the file as long as your lock is in
effect. This facility is available only if the underlying operating system supports file locking.
WARNING
Some operating systems may prevent the use of memory mapping for a file if a
mandatory lock on the file is acquired and vice versa. In such situations using a file lock with
a memory-mapped file does not work.
A lock on a file is encapsulated by an object of the
java.nio.channels.FileLock
class. The
lock()
method for a
FileChannel
object tries to obtain an exclusive lock on the channel's file. Acquiring an ex-
clusive lock on a file ensures that another program cannot access the file at all, and is typically used when
you want to write to a file, or when any modification of the file by another process causes you problems.
Here's one way to obtain an exclusive lock on a file specified by a
Path
object,
file
:
try (FileChannel fileChannel =
(FileChannel)(Files.newByteChannel(file, EnumSet.of(READ, WRITE)));
FileLock fileLock = channel.lock()){
// Work with the locked file...
} catch (IOException e) {
e.printStackTrace();
}
The
lock()
method attempts to acquire an exclusive lock on the channel's entire file so that no other pro-
gram or thread can access the file while this channel holds the lock. A prerequisite for obtaining an exclusive
lock is that the file has been opened for both reading and writing. If another program or thread already has a
lock on the file, the
lock()
method blocks (i.e., does not return) until the lock on the file is released and can
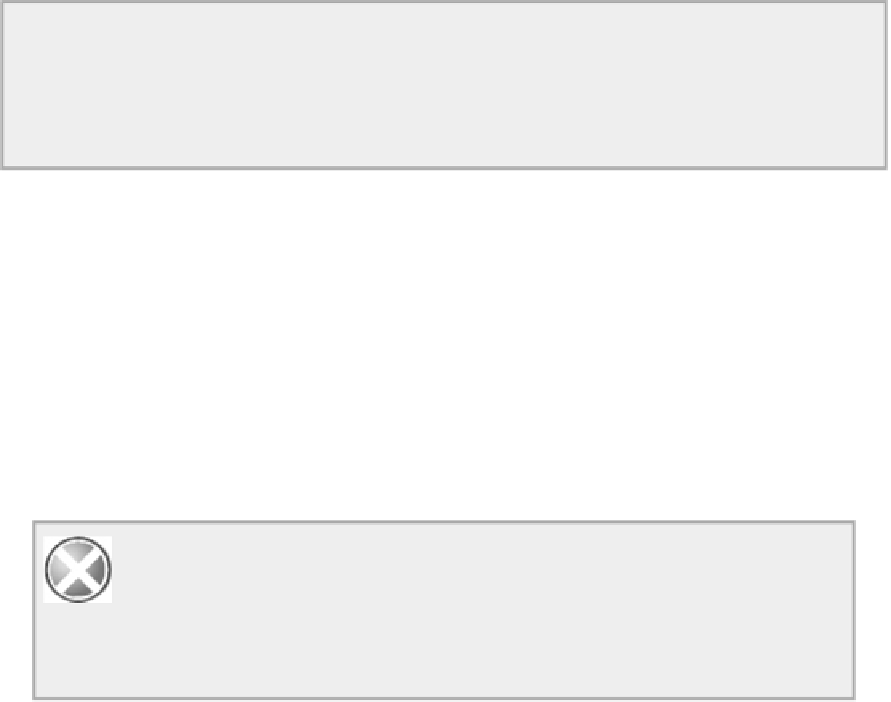