Java Reference
In-Depth Information
}
System.out.println("File written is " +
((FileChannel)channel).size() + "
bytes.");
} catch (IOException e) {
e.printStackTrace();
}
}
PrimesToFile.java
If you don't supply the number of primes you want as a command-line argument, this program produces
the following output:
File written is 800 bytes.
This looks reasonable because you wrote 100 values of type
long
as binary data and they are 8 bytes
each. I ran the program with 150 as the command-line argument.
How It Works
You call the three methods that calculate the primes, create the file path, and write the file in sequence:
getPrimes(primes); // Calculate the
primes
Path file = createFilePath("Beginning Java Stuff",
"primes.bin");
writePrimesFile(primes, file); // Write the file
You could condense the three statements into one:
writePrimesFile(
getPrimes(primes), createFilePath("Beginning Java Stuff",
"primes.bin"));
The references returned by the
getPrimes()
and
createFilePath()
methods are the arguments to the
writePrimesFile()
method. This doesn't improve the readability of the code though, so there's no ad-
vantage gained by this.
The code to calculate the primes and to create a
Path
object for the specified directory and file name in
the user home directory is essentially what you have seen previously so I won't repeat the discussion of
these.
Because you don't specify that you want to append to the file when you create the
channel
object, the
file is overwritten each time you run the program.
You create the
ByteBuffer
object with a capacity of 100 bytes. This is a poor choice for the buffer size as
it is not an exact multiple of 8 — so it doesn't correspond to a whole number of prime values. However,
I chose this value deliberately to make the problem of managing the buffer a bit more interesting. You
can change the buffer size by changing the value specified for
BUFFERSIZE
.
The primes are transferred to the buffer through a view buffer of type
LongBuffer
. You obtain this from
the original byte buffer,
buf
, by calling its
asLongBuffer()
method. Because this buffer is too small to
hold all the primes, you have to load it and write the primes to the file in a loop.
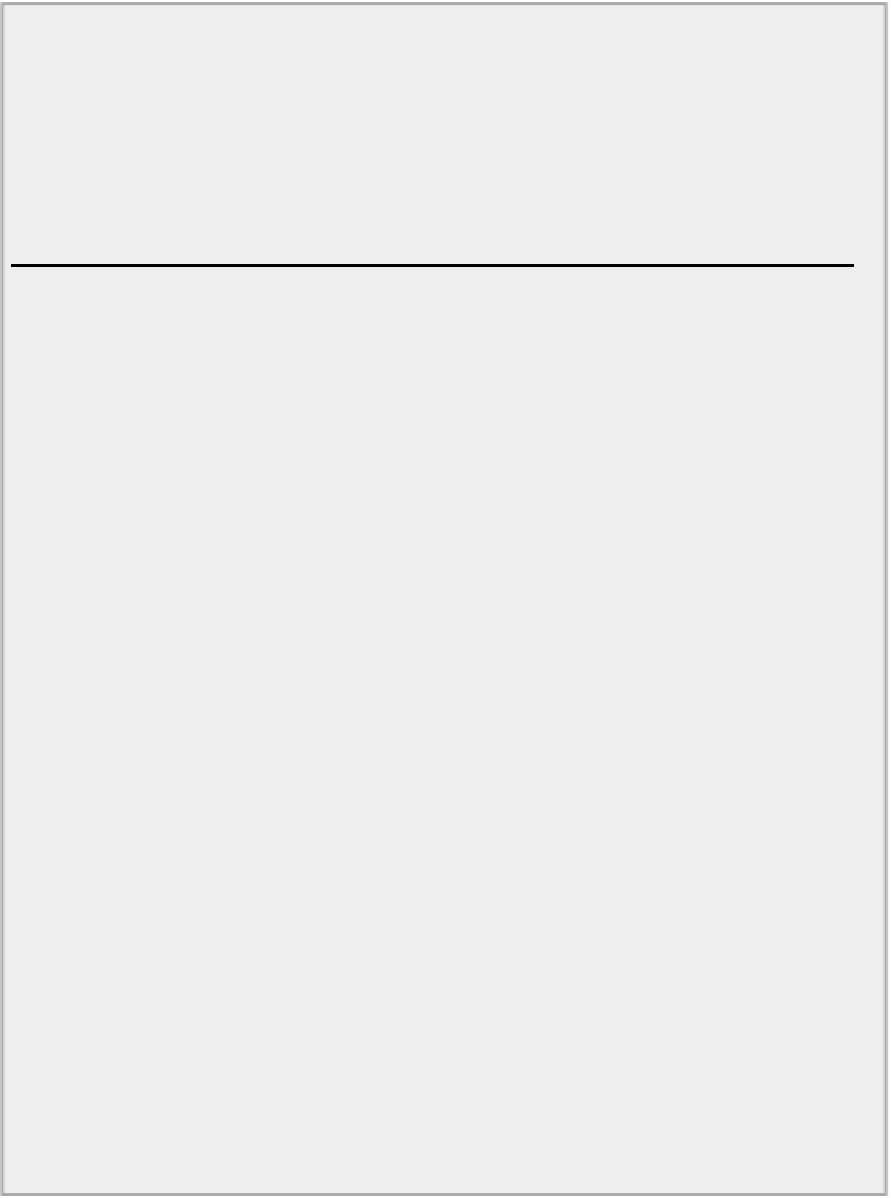
