Java Reference
In-Depth Information
This method copies files from the
from
directory to the
to
directory:
static boolean copyFiles(Path from, Path to) {
if(!isDirectory(from)) {
System.out.println(
"Cannot copy files. " + from + " is not a
directory.");
return false;
} else if(!isDirectory(to)) {
System.out.println("Cannot copy files. " + to + " is not a
directory.");
return false;
}
try (DirectoryStream<Path> files = Files.newDirectoryStream(from,
"*.*")) {
System.out.println("Starting copy...");
for(Path file : files) {
Files.copy(file, to.resolve(file.getFileName()));
// Copy
the file
System.out.println(" " + file.getFileName() + " copied.");
}
} catch(IOException e) {
System.err.println("I/O error in copyFiles. " + e);
return false;
}
return true;
}
MoveAndCopyFiles.java
The parameters for this method are
Path
references specifying the directory that is the source of files to
be copied and the destination directory for the file copies. The method first verifies that both arguments
reference directories using the previous method,
isDirectory()
. Remember that the second argument
to the
copy()
method for a path is a
Path
object referencing the item after the copy has occurred, not
the destination directory. We therefore use the
resolve()
method for the
to
object to combine the des-
tination directory path with the name of the file. The second argument to the
newDirectoryStream()
method is a filter that ensures that the resultant stream only contains files with an extension so directories
excluded. The method outputs the name of each file when it has been copied. The method returns
true
if the copy operation is successful and
false
otherwise.
The method to move files from one directory to another is very similar:
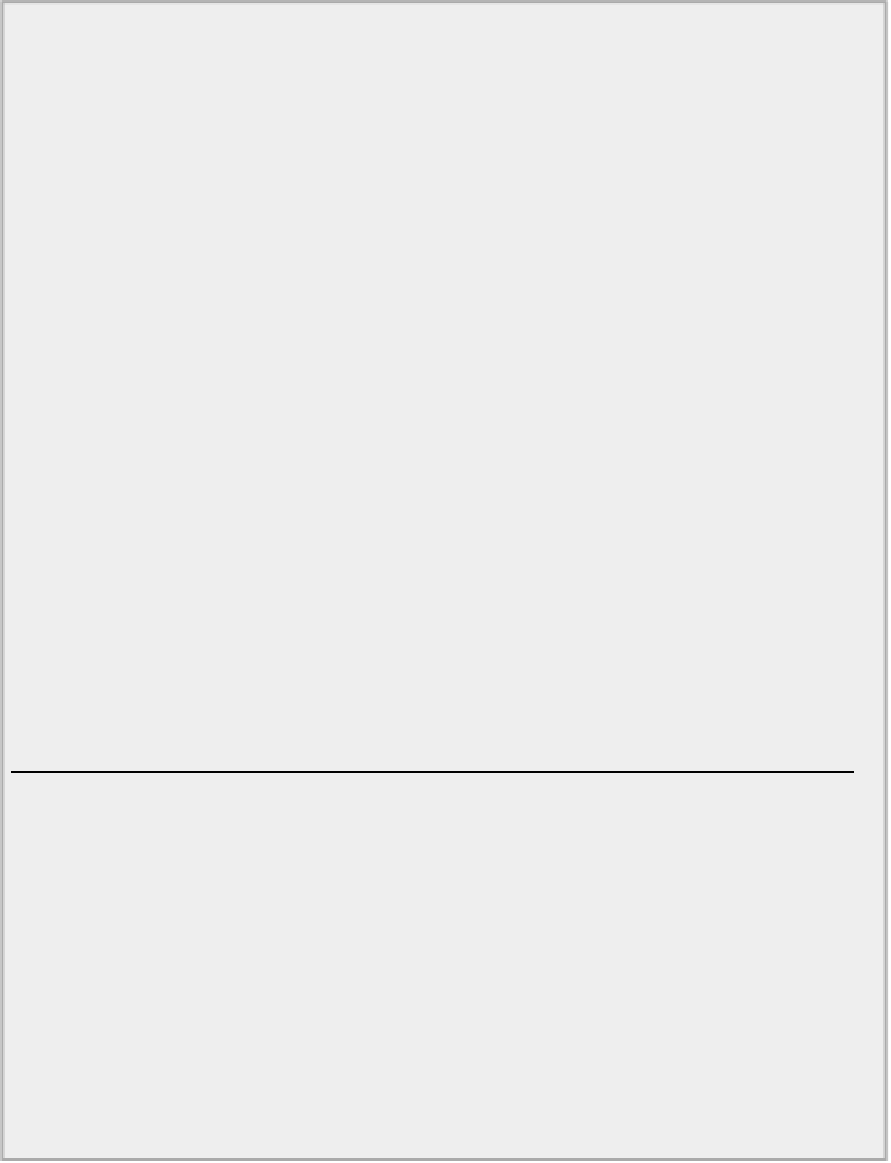


