Java Reference
In-Depth Information
Jacket[] jackets = {new Jacket(JacketSize.medium,
JacketColor.red),
new Jacket(JacketSize.extra_large,
JacketColor.yellow),
new Jacket(JacketSize.small, JacketColor.green),
new Jacket(JacketSize.extra_extra_large,
JacketColor.blue)
};
// Output colors available
System.out.println("Jackets colors available are:\n");
for(JacketColor color: JacketColor.values()) {
System.out.print(" " + color);
}
// Output sizes available
System.out.println("\n\nJackets sizes available are:\n");
for(JacketSize size: JacketSize.values()) {
System.out.print(" " + size);
}
System.out.println("\n\nJackets in stock are:");
for(Jacket jacket: jackets) {
System.out.println(jacket);
}
}
}
Directory "TryJackets"
When you compile and execute this program you get the following output:
Jackets colors available are:
red orange yellow blue green
Jackets sizes available are:
small medium large extra_large extra_extra_large
Jackets in stock are:
Jacket medium in red
Jacket extra_large in yellow
Jacket small in green
Jacket extra_extra_large in blue
How It Works
The
main()
method in the
TryJackets
class defines an array of
Jacket
objects. It then lists the sizes
and colors available for a jacket simply by using the collection-based
for
loop to list the constants
in each enumeration. Because the enumeration constants are objects, the compiler inserts a call to the
toString()
method for the objects to produce the output. You use the same kind of
for
loop to list the
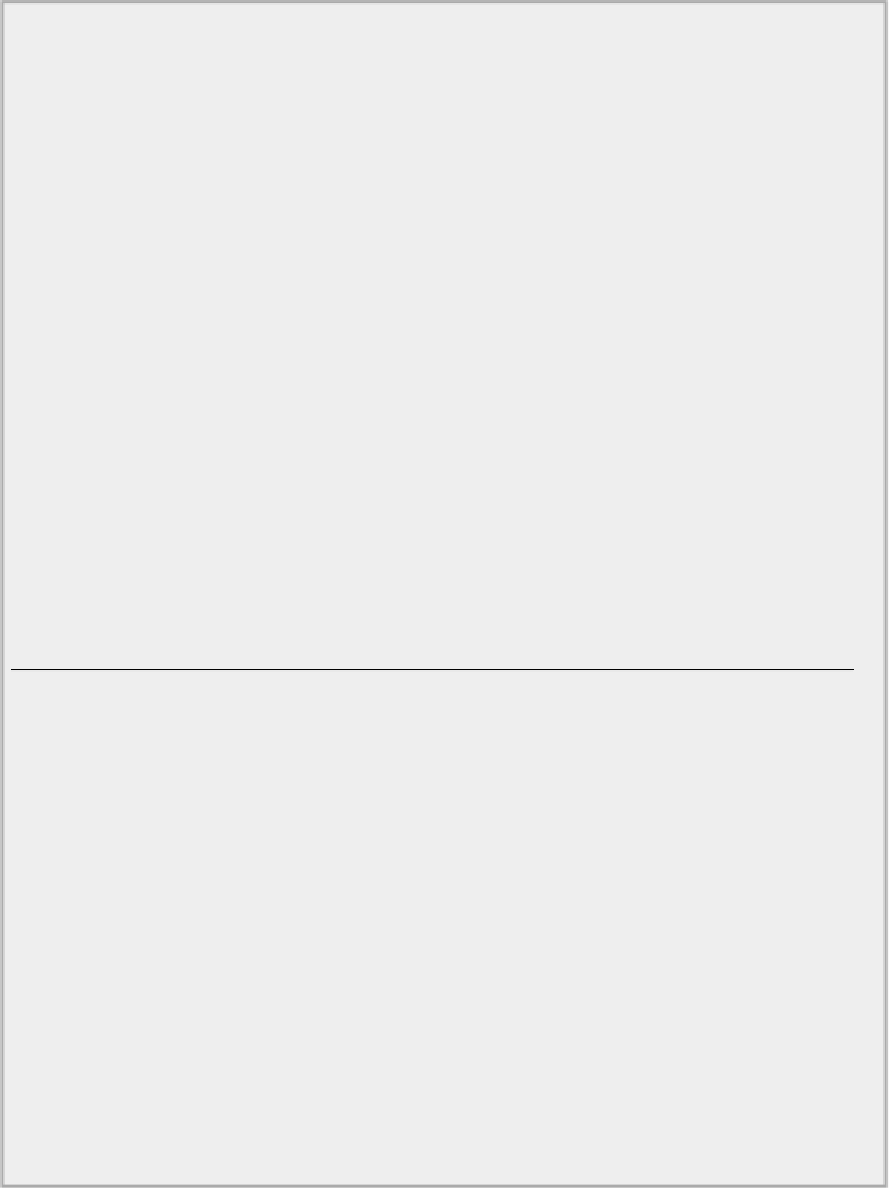
