Java Reference
In-Depth Information
The
Sphere
class definition includes a constructor that creates objects, and the method
volume()
to cal-
culate the volume of a particular sphere. It also contains the
static
method
getCount()
you saw earlier,
which returns the current value of the class variable
count
. You need to define this method as
static
because you want to be able to call it regardless of how many objects have been created, including the
situation when there are none.
The method
main()
in the
CreateSpheres
class puts the
Sphere
class through its paces. When the pro-
gram is compiled, the compiler looks for a file with the name
Sphere.class
. If it does not find the
.class
file, it looks for
Sphere.java
to provide the definition of the class
Sphere
. As long as this file
is in the current directory, the compiler is able to find it and compile it.
The first thing the program does is call the
static
method
getCount()
. Because no objects exist, you
must use the class name to call it at this point. You then create the object
ball
, which is a
Sphere
object,
with a radius of 4.0 and its center at the origin point (0.0, 0.0, 0.0). You call the
getCount()
method
again, this time using the object name. This demonstrates that you can call a
static
method through an
object. You create another
Sphere
object,
globe
, with a radius of 12.0. You call the
getCount()
method
again, this time using the class name. Static methods like this are usually called using the class name.
After all, the reason for calling this particular method is to find out how many objects exist, so presum-
ably you cannot be sure that any objects exist at that point. A further reason to use the class name rather
than a reference to an object when calling a static method is that it makes it quite clear in the source code
that it
is
a static method that is being called. You can't call a non-static method using the class name.
The program finally outputs the volume of both objects by calling the
volume()
method for each, from
within the expressions, specifying the arguments to the
println()
method calls.
METHOD OVERLOADING
Java enables you to define several methods in a class with the same name, as long as each method has a
unique set of parameters. Defining two or more methods with the same name in a class is called
method
overloading
.
The name of a method together with the types and sequence of the parameters form the
signature
of the
method; the signature of each method in a class must be distinct to allow the compiler to determine exactly
which method you are calling at any particular point. The return type has no effect on the signature of a
method. You cannot differentiate between two methods just by the return type. This is because the return
type is not necessarily apparent when you call a method. For example, suppose you write a statement such
as:
Math.round(value);
Although the preceding statement is pointless because it discards the value that the
round()
method pro-
duces, it does illustrate why the return type cannot be part of the signature for a method. The compiler has
no way to know from this statement what the return type of the method
round()
is supposed to be. Thus, if
there were several different versions of the method
round()
, and the return type were the only distinguish-
ing aspect of the method signature, the compiler would be unable to determine which version of
round()
you wanted to use.
You will find many circumstances where it is convenient to use method overloading. You have already
seen that the
Math
class contains two versions of the method
round()
, one that accepts an argument of type
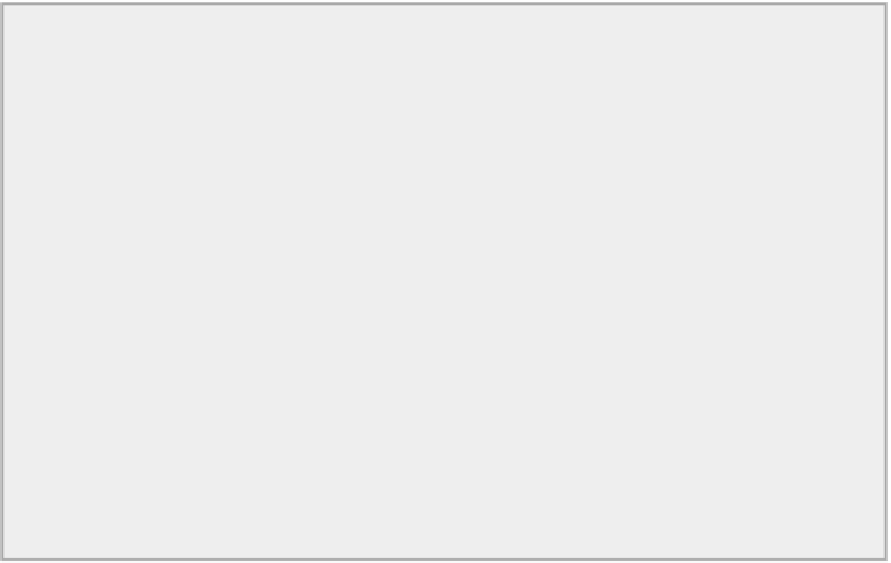