Java Reference
In-Depth Information
NOTE
YoulearnmoreaboutthisinChapter5,whenyoulookathowtodefineyour
own classes. For the moment, just note that you don't necessarily need to pass any
arguments to a method because some methods don't require any arguments. On the
other hand, several arguments can be required. It all depends on how the method
was defined in the class.
The
equals()
method requires one argument that you put between the parentheses. This must be the
String
object that is to be compared with the original object. As you saw earlier, the method returns
true
if the string passed to it (
string3
in the example) is identical to the string pointed to by the
String
object that owns the method; in this case,
string1
. As you also saw in the previous section, you could
just as well call the
equals()
method for the object
string3
, and pass
string1
as the argument to com-
pare the two strings. In this case, the expression to call the method would be
string3.equals(string1)
and you would get exactly the same result.
The statements in the program code after outputting the values of
string3
and
string1
are:
if(string1.equals(string3)) { // Now test for
equality
System.out.println("string1.equals(string3) is true." +
" so strings are equal.");
} else {
System.out.println("string1.equals(string3) is false." +
" so strings are not equal.");
}
The output from this shows that calling the
equals()
method for
string1
with
string3
as the argument
returns
true
. After the
if
statement you make
string3
reference a new string. You then compare the
values of
string1
and
string3
once more, and, of course, the result of the comparison is now
false
.
Finally, you compare
string1
with
string3
using the
equalsIgnoreCase()
method. Here the result is
true
because the strings differ only in the case of the first three characters.
String Interning
Having convinced you of the necessity for using the
equals
method for comparing strings, I can now reveal
that there is a way to make comparing strings with the
==
operator effective. The mechanism to make this
possible is called
string interning
. String interning ensures that no two
String
objects encapsulate the same
string, so all
String
objects encapsulate unique strings. This means that if two
String
variables reference
strings that are identical, the references must be identical, too. To put it another way, if two
String
variables
contain references that are not equal, they must refer to strings that are different. So how do you arrange that
all
String
objects encapsulate unique strings? You just call the
intern()
method for every new
String
object that you create. To show how this works, I can amend a bit of an earlier example:
String string1 = "Too many ";
String string2 = "cooks";
String string3 = "Too many cooks";
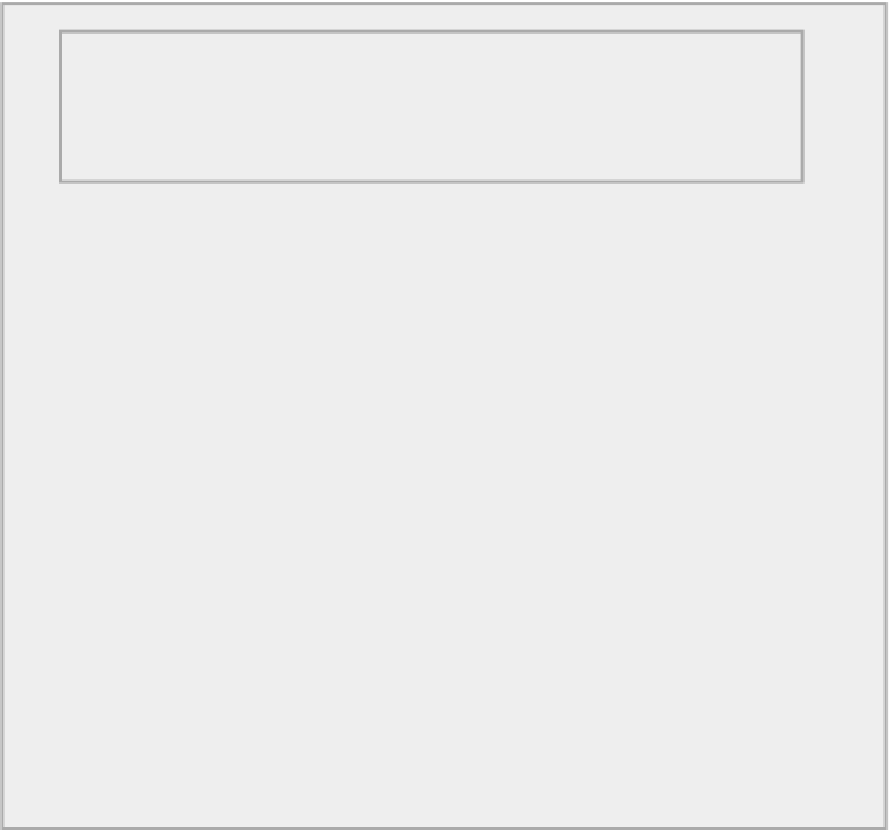