Java Reference
In-Depth Information
sion between the
for
loop parentheses to increment
number
in steps of two because you don't want to
check even numbers. The
for
loop ends when
count
is equal to the length of the array. You test the value
in
number
in the inner
for
loop by dividing
number
by all of the prime numbers you have in the
primes
array that are less than, or equal to, the square root of the candidate. If you get an exact division, the value
in
number
is not prime, so you go immediately to the next iteration of the
outer
loop via the
continue
statement.
You calculate the limit for divisors you need to try with the following statement:
long limit = (long)ceil(sqrt((double)number));
The
sqrt()
method from the
Math
class produces the square root of
number
as a
double
value, so if
number
has the value 7, for example, a value of about 2.64575 is returned. This is passed to the
ceil()
method, which is also a member of the
Math
class. The
ceil()
method returns a value of type
double
that is the minimum whole number that is not less than the value passed to it. With
number
as 7, this
returns 3.0, the smallest integral value not less than the square root of 7. You want to use this number
as the limit for your integer divisors, so you cast it to type
long
and store the result in
limit
. You are
able to call the
sqrt()
and
ceil()
methods without qualifying their names with the class to which they
belong because you have imported their names into the source file.
The cast of
number
to type
double
is not strictly necessary. You could write the statement as:
long limit = (long)ceil(sqrt(number));
The compiler will insert the cast for you. However, by putting the explicit cast in, you indicate that it was
your intention.
If you don't get an exact division, you exit normally from the inner loop and execute the statement:
primes[count++] = number; // We got one!
Because
count
is the number of values you have stored, it also corresponds to the index for the next free
element in the
primes
array. Thus, you use
count
as the index to the array element in which you want to
store the value of
number
and then increment
count
.
When you have filled the
primes
array, the
outer
loop ends and you output all the values in the array in
the loop:
for(long n : primes) {
System.out.println(n); // Output all the primes
}
This loop iterates through all the elements of type
long
in the
primes
array in sequence. On each itera-
tion
n
contains the value of the current element, so that is written out by the
println()
method.
You can express the logical process of this program as the following sequence of steps:
1.
Take the
number
in question and determine its square root.
2.
Set the
limit
for divisors to be the smallest integer that is greater than this square root value.
3.
Test to see if the
number
can be divided exactly (without remainder) by any of the
primes
already
in the
primes
array
that are less than the
limit
for divisors.
4.
If any of the existing primes divide into the current
number
, discard the current
number
and start
a new iteration of the loop with the next candidate
number
.
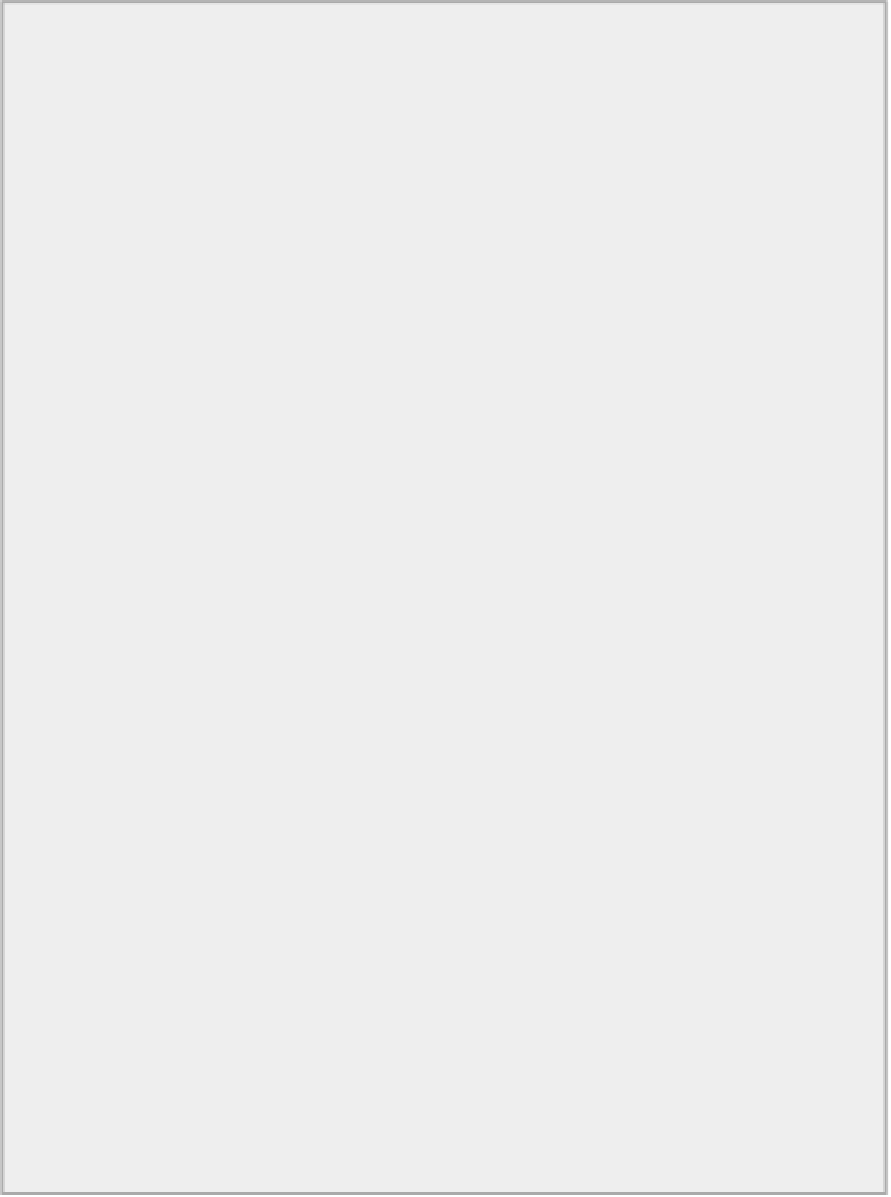