Java Reference
In-Depth Information
temp = inputArray; // Save reference to inputArray in temp
inputArray = outputArray; // Set inputArray to refer to outputArray
outputArray = temp; // Set outputArray to refer to what was inputArray
None of the array elements are moved here. Just the addresses of where the arrays are located in memory
are swapped, so this is a very fast process. Of course, if you want to replicate an array, you have to create a
new array of the same size and type, and then copy the value of each element of the old array to your new
array.
NOTE
I'm sure that you realize that you can copy the contents of an array to a new array
usingaloop.InChapter15youlearnaboutmethodsinthe
Arrays
classthatcandothismore
efficiently.
Using Arrays
You can use array elements in expressions in exactly the same way as you might use a single variable of the
same data type. For example, if you declare an array
samples
, you can fill it with random values between
0.0 and 100.0 with the following code:
double[] samples = new double[50]; // An array of 50 double values
for(int i = 0; i < samples.length; ++i) {
samples[i] = 100.0*Math.random(); // Generate random values
}
This shows how the numerical
for
loop is ideal when you want to iterate through the elements in an array
to set their values. Of course, this is not an accident. A major reason for the existence of the
for
loop is
precisely for iterating over the elements in an array.
To show that array elements can be used in exactly the same way as ordinary variables, I could write the
following statement:
double result = (samples[10]*samples[0] - Math.sqrt(samples[49]))/samples[29];
This is a totally arbitrary calculation, of course. More sensibly, to compute the average of the values
stored in the
samples
array, you could write
double average = 0.0; // Variable to hold the average
for(int i = 0; i < samples.length; ++i) {
average += samples[i]; // Sum all the elements
}
average /= samples.length; // Divide by the total number of elements
Within the loop, you accumulate the sum of all the elements of the array
samples
in the variable
aver-
age
. You then divide this sum by the number of elements.
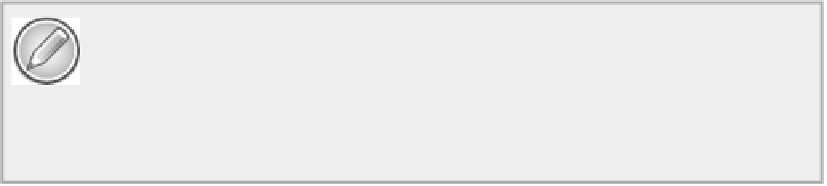