Java Reference
In-Depth Information
There are often several ways of writing the code to produce a given result, and this is true here — you
could also move the incrementing of the variable
i
back inside the loop and write it as follows:
do {
sum += i++; // Add the current value of
i to sum
} while (i <= limit);
The value of
i
is now incremented using the postfix increment operator. If you were to use the prefix
form, you would get the wrong result. Note that the semicolon after the
while
condition is present in
each version of the loop. This is part of the loop statement so you must not forget to put it in. The primary
reason for using this loop over the
while
loop is if you want to be sure that the loop code always executes
at least once.
Counting Using Floating-Point Values
You can use a floating-point variable as the loop counter in a
for
loop if you need to. This may be needed
when you are calculating the value of a function for a range of fractional values. Suppose you want to cal-
culate the area of a circle with values for the radius from 1 to 2 in steps of 0.2. You could write this as
for(double radius = 1.0 ; radius <= 2.0 ; radius += 0.2) {
System.out.println("radius = " + radius + " area = " + Math.PI*radius*radius);
}
which produces the following output:
radius = 1.0 area = 3.141592653589793
radius = 1.2 area = 4.523893421169302
radius = 1.4 area = 6.157521601035994
radius = 1.5999999999999999 area = 8.04247719318987
radius = 1.7999999999999998 area = 10.178760197630927
radius = 1.9999999999999998 area = 12.566370614359169
The area has been calculated using the formula πr
2
with the standard value
PI
defined in the
Math
class,
which is 3.14159265358979323846. Although you may have intended the values of
radius
to increment
from 1.0 to 2.0 in steps of 0.2, they don't quite make it. The value of radius is never exactly 2.0 or any of
the other intermediate values because 0.2 cannot be represented exactly as a binary floating-point value. If
you doubt this, and you are prepared to deal with an infinite loop, change the loop to the following:
// BE WARNED - THIS LOOP DOES NOT END
for(double radius = 1.0; radius != 2.0; radius += 0.2) {
System.out.println("radius = " + radius + " area = " + Math.PI*radius*radius);
}
If the value of
radius
reaches 2.0, the condition
radius != 2.0
is
false
and the loop ends, but unfor-
tunately, it doesn't. Its last value before 2 is approximately 1.999 . . . and the next value is something like
2.1999 . . . and so it is never 2.0. From this you can deduce a golden rule:
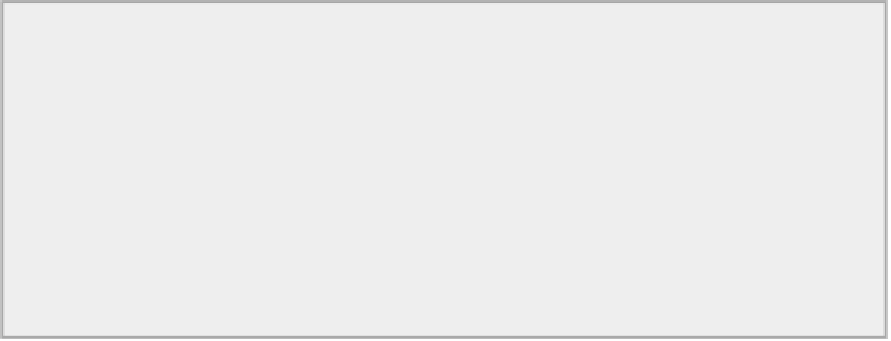