Java Reference
In-Depth Information
How It Works
This is stuff that should be very familiar to you by now. You create a
JMenu
object for the Help item on
the menu bar, and add a shortcut for it by calling its
setMnemonic()
member. You create a
JMenuItem
object, which is the About menu item, and call its
addActionListener()
method to make the
Sketch-
erFrame
object the listener for the item. After adding the menu item to the Help menu, you add the
helpMenu
object to the
menuBar
object.
You create an
AboutDialog
object in the
actionPerformed()
method for the
SketcherFrame
object,
as this method is called when the About menu item is clicked. Before you display the dialog, you verify
that the source of the event is the menu item,
aboutItem
. This is not important now, but you will add
other menu items later, and you will handle their events using the same
actionPerformed()
method.
The dialog object is self-contained and disposes of itself when the OK button is clicked. The dialog that
you want to display here always displays the same message, so there's no real point in creating and des-
troying it each time you want to display it. You could arrange for the dialog box object to be created
once and the reference stored as a member of the
SketcherFrame
class. Then you could make it visible
in the
actionPerformed()
method for the menu item and make it invisible in the
actionPerformed()
method responding to the dialog OK button event.
This is all very well, but it was a lot of work just to get a dialog with a message displayed. Deriving a
class from
JDialog
gives you complete flexibility as to how the dialog works, but you didn't really need
it in this case. Didn't I say there was an easier way?
Instant Message Dialogs
The
JOptionPane
class in the
javax.swing
package defines static methods that create and display standard
modal dialogs for you. The simplest dialog you can create this way is a message dialog rather like the About
message dialog in the previous example. The static methods in the
JOptionPane
class, shown in
Table 20-1
,
produce message dialogs:
DESCRIPTION
This displays a modal dialog with the default title
"Message"
. The first argument is the parent
for the dialog that is used to position the dialog. If the first argument is
null
, a default
Frame
object is created and used to position the dialog centrally on the screen.The second argument
specifies what is to be displayed in addition to the default OK button. This can be a
String
ob-
ject specifying the message, an
Icon
object defining an icon to be displayed, or a
Component
reference to an object. If you pass some other type of object reference as the second argument,
then its
toString()
method is called, and the
String
object that is returned is displayed. You
usually get a default icon for an information message along with your message.
showMessageDialog(
Component parent,
Object message)
This displays a dialog just as the first method in this table, but with the title specified by the
third argument. The fourth argument,
messageType
, can be:
ERROR_MESSAGE
INFORMATION_MESSAGE WARNING_MESSAGE QUESTION_MESSAGE PLAIN_MESSAGE
These static
JOptionPane
fields determine the style of the message constrained by the current look-and-feel.
This usually includes a default icon, such as a question mark for
QUESTION_MESSAGE
.
showMessageDialog(
Component parent,
Object message,
String title, int
messageType)
This displays a dialog as the second method in this table, except that the icon is what you pass
as the fifth argument. Specifying a
null
icon argument produces a dialog just as the second
method in this table.
showMessageDialog(
Component parent,
Object message,
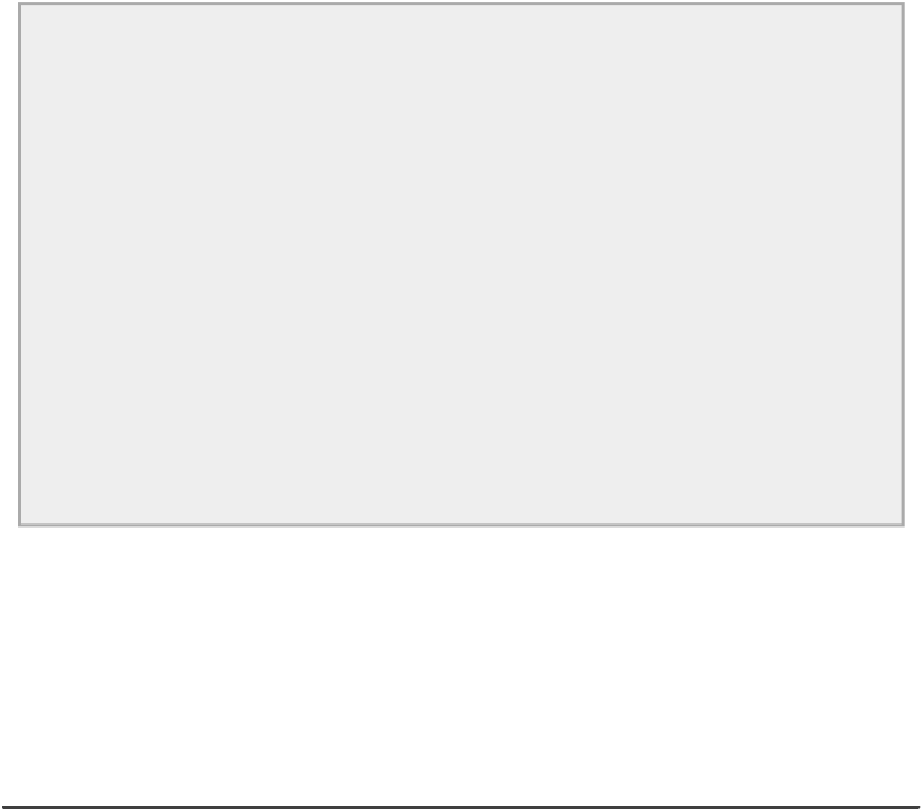





