Java Reference
In-Depth Information
Display 15.7
A Linked List Class with a Node Inner Class
(part 2 of 3)
12
public
Node(String newItem, Node linkValue)
13 {
14 item = newItem;
15 link = linkValue;
16 }
17 }
//End of Node inner class
18
private
Node head;
19
public
LinkedList2( )
20 {
21 head =
null
;
22 }
23
/**
24
Adds a node at the start of the list with the specified data.
25
The added node will be the first node in the list.
26
*/
27
public void
addToStart(String itemName)
28 {
29 head =
new
Node(itemName, head);
30 }
31
/**
32
Removes the head node and returns true if the list contains at
33
least one node. Returns false if the list is empty.
34
*/
35
public boolean
deleteHeadNode( )
36 {
37
if
(head !=
null
)
38 {
39 head = head.link;
40
return true
;
41 }
42 else
43
return false
;
44 }
45
/**
46
Returns the number of nodes in the list.
47
*/
48
public int
size( )
49 {
50
int
count = 0;
51 Node position = head;
52
while
(position !=
null
)
53 {
54 count++;
(continued)
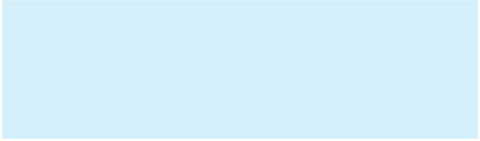
