Java Reference
In-Depth Information
Programming Projects
Visit
www.myprogramminglab.com
to complete select exercises online and get instant
feedback.
1. A savings account typically accrues savings using compound interest. If you deposit
$1,000 with a 10% interest rate per year, then after one year you have a total of
$1,100. If you leave this money in the account for another year at 10% interest,
then after two years the total will be $1,210. After three years, you would have
$1,331, and so on.
Write a program that inputs the amount of money to deposit, an interest rate per
year, and the number of years the money will accrue compound interest. Write a
recursive function that calculates the amount of money that will be in the savings
account using the input information.
To verify your function, the amount should be equal to
P(
1+
i)
n
, where
P
is the
amount initially saved,
i
is the interest rate per year, and
n
is the number of years.
2. There are
n
people in a room, where
n
is an integer greater than or equal to 1. Each
person shakes hands once with every other person. What is the total number,
h(n)
,
of handshakes? Write a recursive function to solve this problem. To get you started,
if there are only one or two people in the room, then
handshake(1) = 0
handshake(2) = 1
If a third person enters the room, he or she must shake hands with each of the
two people already there. This is two handshakes in addition to the number
of handshakes that would be made in a room of two people, or a total of three
handshakes.
If a fourth person enters the room, he or she must shake hands with each of the
three people already present. This is three handshakes in addition to the number
of handshakes that would be made in a room of three people, or six handshakes.
If you can generalize this to
n
handshakes, then it should help you write the
recursive solution.
3. Consider a frame of bowling pins shown below, where each
*
represents a pin:
*
* *
* * *
* * * *
* * * * *
There are 5 rows and a total of 15 pins.
If we had only the top 4 rows, then there would be a total of 10 pins.
If we had only the top three rows, then there would be a total of six pins.
If we had only the top two rows, then there would be a total of three pins.
If we had only the top row, then there would be a total of one pin.
VideoNote
Solution to
Programming
Project 11.3
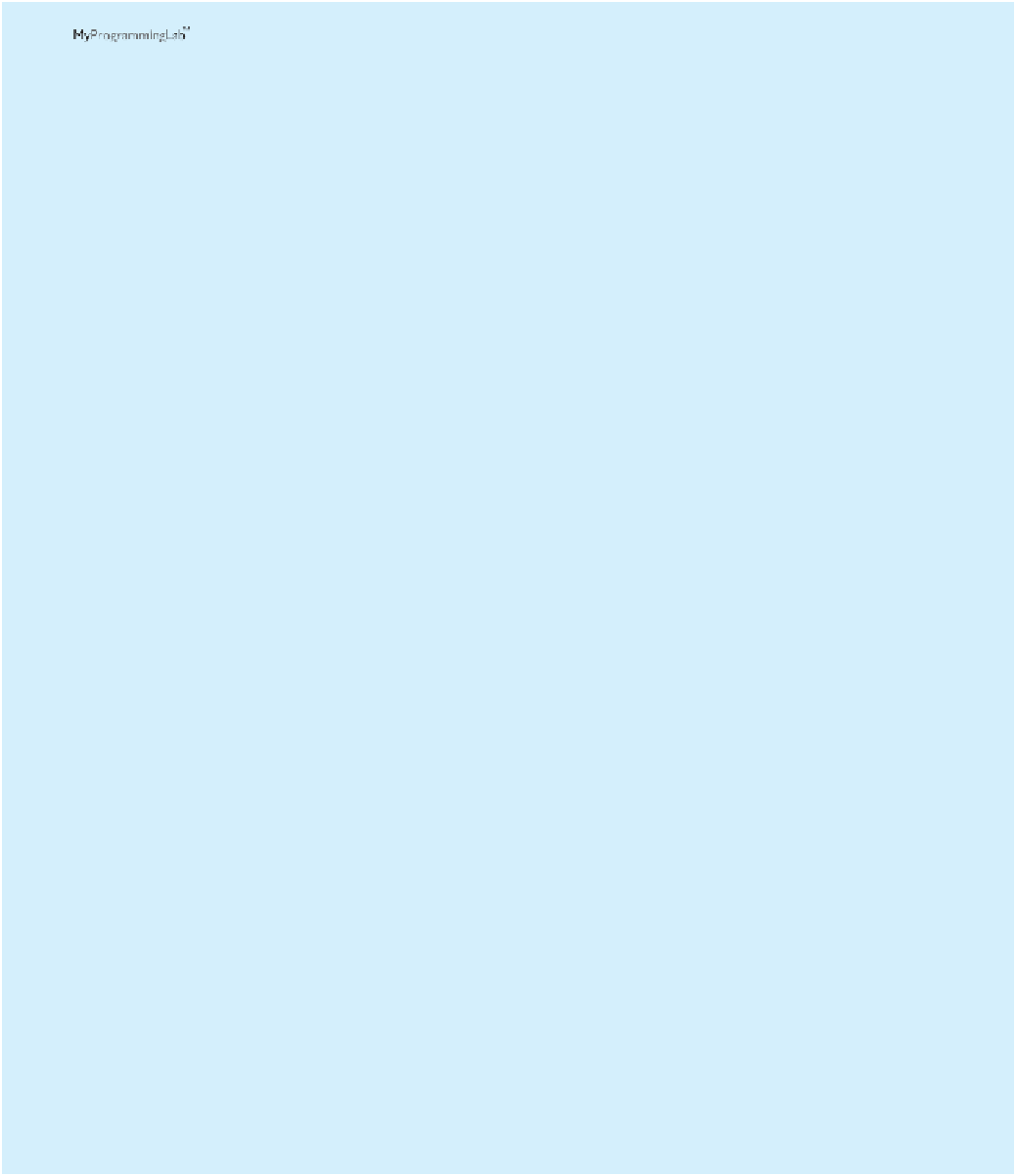








