Java Reference
In-Depth Information
EXAMPLE:
(continued)
The task to be performed by
writeVertical
may be broken down into the following
two subtasks:
Simple Case:
If
n < 10
, then write the number
n
to the screen.
After all, if the number is only one digit long, the task is trivial.
Recursive Case:
If
n >= 10
, then do two subtasks:
1. Output all the digits except the last digit.
2. Output the last digit.
For example, if the argument were
1234
, the first part would output
1
2
3
and the second part would output
4
. This decomposition of tasks into subtasks can
be used to derive the method definition.
Subtask 1 is a smaller version of the original task, so we can implement this
subtask with a recursive call. Subtask 2 is just the simple case we listed previously.
Thus, an outline of our algorithm for the method
writeVertical
with parameter
n
is given by the following pseudocode:
if
(n < 10)
{
System.out.println(n);
}
else
//n is two or more digits long:
{
writeVertical(
the number
n
with the last digit removed
);
System.out.println(
the last digit of
n);
}
Recursive subtask
If you observe the following identities, it is easy to convert this pseudocode to a
complete Java method definition:
n / 10
is the number
n
with the last digit removed.
n % 10
is the last digit of
n
.
For example,
1234 / 10
evaluates to
123
and
1234 % 10
evaluates to
4
.
(continued)
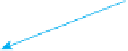