Java Reference
In-Depth Information
the file, or read from the file, is not just bytes but also strings or items of any of Java's
primitive data types, such as
int
,
char
, and
double
, or even objects of classes you
define. If you do not need to access your files using an editor, then the easiest and most
efficient way to read data from and write data to files is to use binary files in the way we
describe here.
We conclude this section with a discussion of how you can use
ObjectOutputStream
and
ObjectInputStream
to write and later read objects of any class you define. This
will let you store objects of the classes you define in binary files and later read them
back, all with the same convenience and efficiency that you get when storing strings
and primitive type data in binary files.
Writing Simple Data to a Binary File
The class
ObjectOutputStream
is the preferred stream class for writing to a binary
file.
2
An object of the class
ObjectOutputStream
has methods to write strings and
values of any of the primitive types to a binary file. Display 10.13 shows a sample
program that writes values of type
int
to a binary file. Display 10.14 describes the
methods used for writing data of other types to a binary file.
Display 10.13
Writing to a Binary File
(part 1 of 2)
1
import
java.io.ObjectOutputStream;
2
import
java.io.FileOutputStream;
3
import
java.io.IOException;
4
public class
BinaryOutputDemo
5 {
6
public static void
main(String[] args)
7 {
8
try
9 {
10 ObjectOutputStream outputStream =
11
new
ObjectOutputStream(
new
FileOutputStream("numbers.dat"));
12
int
i;
13
for
(i = 0; i < 5; i++)
14 outputStream.writeInt(i);
15 System.out.println("Numbers written to the file numbers.dat.");
16 outputStream.close();
17 }
2
DataOutputStream
is also widely used and behaves exactly as we describe for
ObjectOutputStream
in this section. However, the techniques given in the subsections “Binary I/O of Objects” and “Array Objects
in Binary Files” work only for
ObjectOutputStream
; they do not work for
DataOutputStream
.
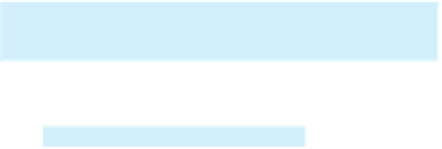
