Java Reference
In-Depth Information
Display 9.1
An Exception Controlled Loop
1
import
java.util.Scanner;
2
import
java.util.InputMismatchException;
3
public class
InputMismatchExceptionDemo
4 {
5
public static void
main(String[] args)
6 {
7 Scanner keyboard =
new
Scanner(System.in);
8
int
number = 0; //
to keep compiler happy
9
boolean
done =
false;
10
while
(! done)
11 {
12
try
13 {
14 System.out.println("Enter a whole number:");
15 number = keyboard.nextInt();
16 done =
true
;
17 }
18
catch
(InputMismatchException e)
19 {
20 keyboard.nextLine();
21 System.out.println("Not a correctly written whole
number.");
22 System.out.println("Try again.");
23 }
24 }
If
nextInt
throws an exception, the
try
block ends and the
Boolean
variable
done
is not set to
true
.
25 System.out.println("You entered " + number);
26 }
27 }
Sample Dialogue
Enter a whole number:
forty two
Not a correctly written whole number.
Try again.
Enter a whole number:
Fortytwo
Not a correctly written whole number.
Try again.
Enter a whole number:
42
You entered 42


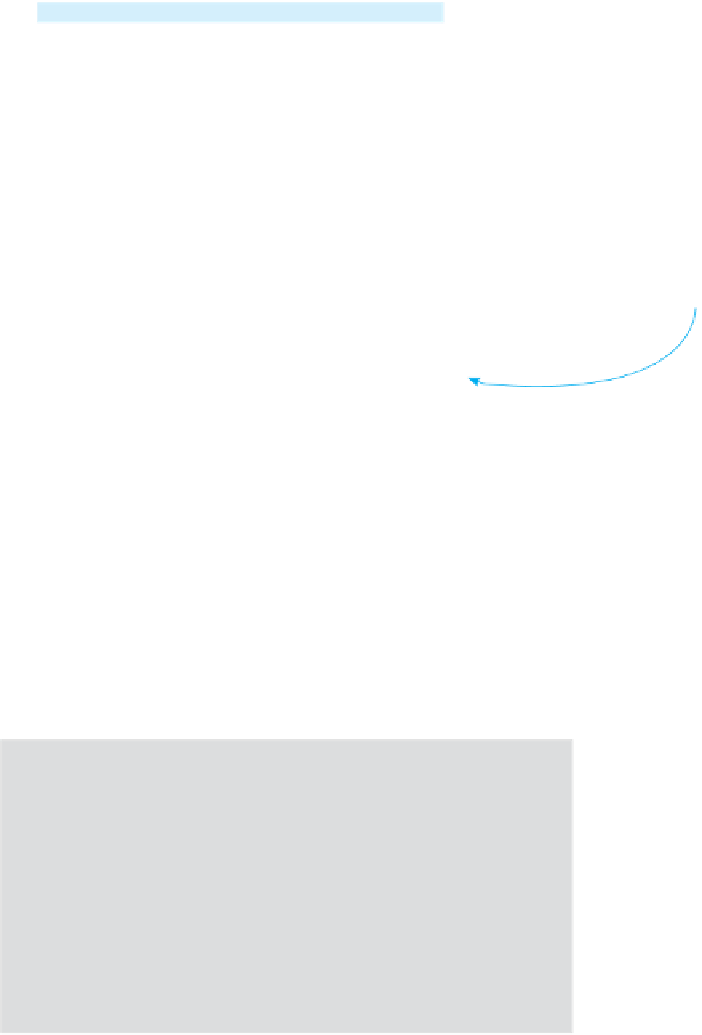

