Java Reference
In-Depth Information
4. Define a class called
BlogEntry
that could be used to store an entry for a Web
log. The class should have instance variables to store the poster's username, text of
the entry, and the date of the entry using the
Date
class from this chapter. Add a
constructor that allows the user of the class to set all instance variables. Also add
a method,
DisplayEntry
, that outputs all of the instance variables, and another
method called
getSummary
that returns the first 10 words from the text (or the
entire text if it is less than 10 words). Test your class from your main method.
5. Define a class called
Counter
whose objects count things. An object of this class
records a count that is a nonnegative integer. Include methods to set the counter
to 0, to increase the count by 1, and to decrease the count by 1. Be sure that no
method allows the value of the counter to become negative. Include an accessor
method that returns the current count value and a method that outputs the count
to the screen. There should be no input method or other mutator methods. The
only method that can set the counter is the one that sets it to 0. Also, include a
toString
method and an
equals
method. Write a program (or programs) to test
all the methods in your class definition.
6. Write a grading program for a class with the following grading policies:
a. There are three quizzes, each graded on the basis of 10 points.
b. There is one midterm exam, graded on the basis of 100 points.
c. There is one final exam, graded on the basis of 100 points.
The final exam counts for 40% of the grade. The midterm counts for 35% of the
grade. The three quizzes together count for a total of 25% of the grade. (Do not
forget to convert the quiz scores to percentages before they are averaged in.)
Any grade of 90 or more is an A, any grade of 80 or more (but less than 90) is a
B, any grade of 70 or more (but less than 80) is a C, any grade of 60 or more (but
less than 70) is a D, and any grade below 60 is an F. The program should read in
the student's scores and output the student's record, which consists of three quiz
scores and two exam scores, as well as the student's overall numeric score for the
entire course and final letter grade.
Define and use a class for the student record. The class should have instance vari-
ables for the quizzes, midterm, final, overall numeric score for the course, and
final letter grade. The overall numeric score is a number in the range 0 to 100,
which represents the weighted average of the student's work. The class should have
methods to compute the overall numeric grade and the final letter grade. These last
methods should be
void
methods that set the appropriate instance variables. Your
class should have a reasonable set of accessor and mutator methods, an
equals
method, and a
toString
method, whether or not your program uses them. You
may add other methods if you wish.
7. Write a
Temperature
class that has two instance variables: a temperature value
(a floating-point number) and a character for the scale, either
C
for Celsius or
F
for
Fahrenheit. The class should have four constructor methods: one for each instance
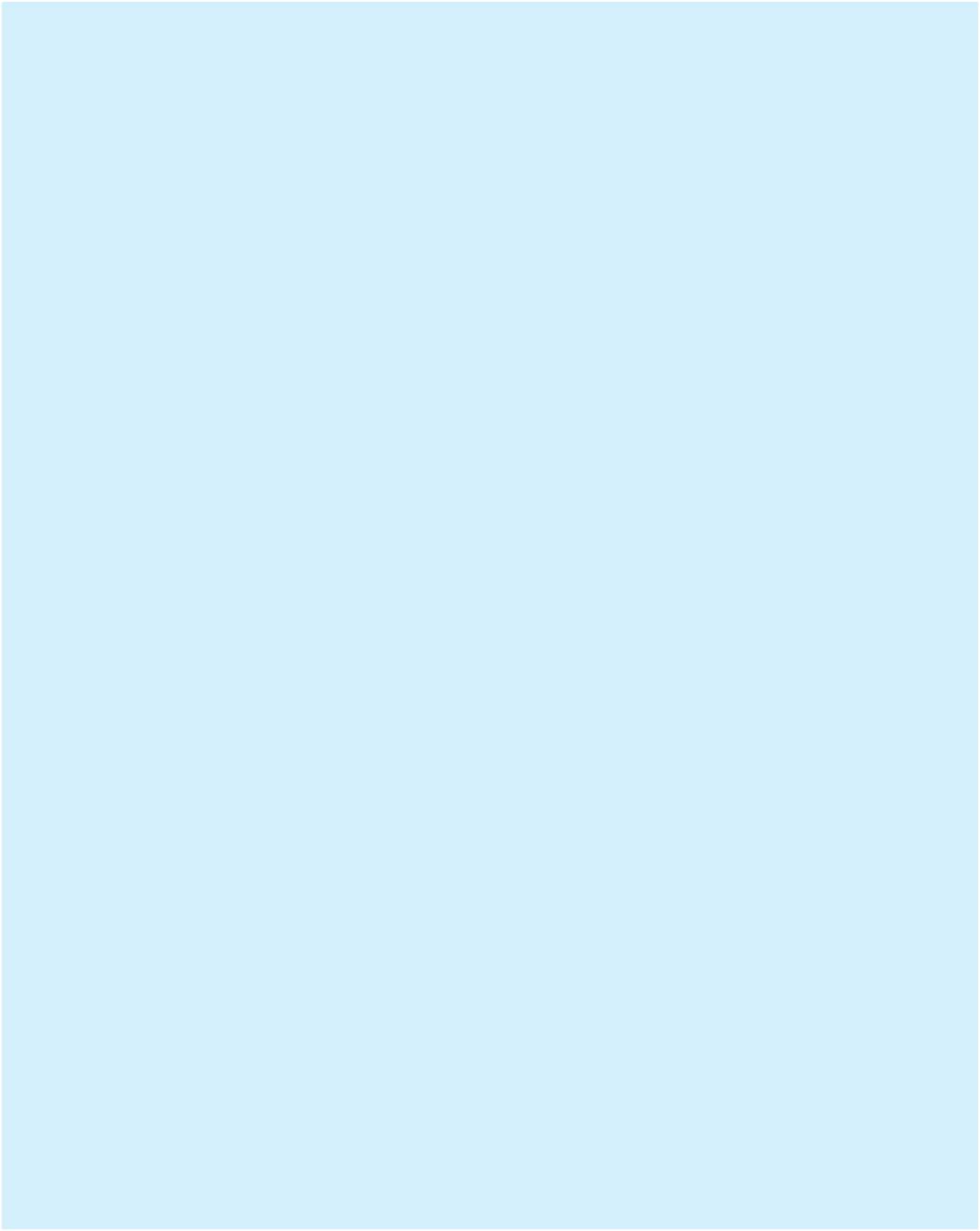