Java Reference
In-Depth Information
EXAMPLE:
(continued)
(the entire GUI) is the listener, not the
JPanel.
So when you click one of the
buttons, it is the
actionPerformed
method in
PanelDemo
that is executed.
When a button is clicked, the
actionPerformed
method is invoked with the action
event fired as the argument to
actionPerformed.
The method
actionPerformed
recovers the string written on the button with the following line:
String buttonString = e.getActionCommand();
The method
actionPerformed
then uses a multiway
if-else
statement to
determine if
buttonString
is
"Blue"
,
"White"
, or
"Gray"
and changes the color of
the corresponding panel accordingly. It is common for an
actionPerformed
method
to be based on such a multiway
if-else
statement, although we will see another
approach in the subsection entitled “Listeners as Inner Classes” later in this chapter.
Display 17.11 also introduces one other small but new technique. We gave
each button a color. We did this with the method
setBackground
, using basically
the same technique that we used in previous examples. You can give a button or
almost any other item a color using
setBackground.
Note that you do not use
getContentPane
when adding color to any component other than a
JFrame
.
Display 17.11
Using Panels
(part 1 of 4)
In addition to being the GUI class, the
class
PanelDemo
is the action listener
class. An object of the class
PanelDemo
is the action listener for the buttons in
that object.
1
import
javax.swing.JFrame;
2
import
javax.swing.JPanel;
3
import
java.awt.BorderLayout;
4
import
java.awt.GridLayout;
5
import
java.awt.FlowLayout;
6
import
java.awt.Color;
7
import
javax.swing.JButton;
8
import
java.awt.event.ActionListener;
9
import
java.awt.event.ActionEvent;
10
public class
PanelDemo
extends
JFrame
implements
ActionListener
11 {
12
public static final int
WIDTH = 300;
13
public static final int
HEIGHT = 200;
We made these instance variables
because we want to refer to them in
both the constructor and the method
actionPerformed
.
14
private
JPanel bluePanel;
15
private
JPanel whitePanel;
16
private
JPanel grayPanel;
17
public static void
main(String[] args)
18 {
19 PanelDemo gui =
new
PanelDemo();
20 gui.setVisible(true);
21 }
(continued)
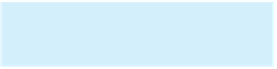



