Hardware Reference
In-Depth Information
2.
Open IDLE, the Python Integrated Development Environment (IDE). This is
your window into the Python programming language and where you type and
run all your Python programs.
3.
Click File
➪
New File from the menu. Save this program by clicking File
➪
Save As
and name the file
block.py
. Remember to store your programs inside the
MyAdventures
folder, otherwise they will not work.
4.
Now, import the modules you need for this adventure. You'll be using an extra
module called
block
, which holds all the constant numbers for all the block
types that Minecraft supports. Do this by typing:
import mcpi.minecraft as minecraft
import mcpi.block as block
5.
Connect to the Minecraft game by typing:
mc = minecraft.Minecraft.create()
6.
Get your player's position into the
pos
variable. You will use this position to
calculate the coordinates of a space in front of your player, where you are going
to create your new block:
pos = mc.player.getTilePos()
7.
Now create a block in front of the player, using coordinates that are relative to
your player's position. You can read all about
relative coordinates
after this sec-
tion. By using
pos+3
in your program it ensures that the stone block doesn't
appear right on top of your player! Do this by typing:
mc.setBlock(pos.x+3, pos.y, pos.z, block.STONE.id)
8.
Click File
➪
Save to save your program and then run it by choosing Run
➪
Run
Module from the editor menu.
You should now see a block of stone very close to your player! You have just pro-
grammed the Minecraft world to create a block in front of you. From these simple
beginnings, you can now create some really interesting structures automatically by
programming Minecraft.
CHALLENGE
Stone isn't a very interesting block. Look at the blocks in Appendix B and experi-
ment by changing the
STONE
in this program to other blocks. Some of the blocks
don't work on all platforms, so Appendix B lists which platforms they work on.
GLOWING_OBSIDIAN
for example works on the Raspberry Pi, but not on the
PC/Mac versions. Some blocks are affected by gravity, so experiment with
WATER
and
SAND
for some interesting effects!































































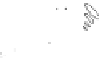
















































