Hardware Reference
In-Depth Information
2.
Create a new empty list, and show what is in the list:
a = [] # an empty list
print(a)
3.
Add a new item to the list, and print it again:
a.append("hello")
print(a)
4.
Add a second item to the list, and print it again:
a.append("minecraft")
print(a)
5.
Check how long the list is:
print(len(a))
6.
Look at items at specific positions within the list. These positions are called
indexes
:
print(a[0]) # the [0] is the index of the first item
print(a[1]) # the [1] is the index of the second item
7.
Remove one word from the end of the list, and show that word and the remain-
ing list:
word = a.pop()
print(word)
print(a)
8.
Check how long the list is, and check how long the word string is:
print(len(a))
print(len(word))
9.
Remove the final item from the list, show the item and the list, and show the
length of the list:
word = a.pop()
print(word)
print(a)
print(len(a))
FigureĀ 4-3 shows the output of these experiments in the Python Shell.
An
index
is a number that identifies which item in a list of items to access. You
specify the index in square brackets like
a[0]
for the first item and
a[1]
for the
second item. Indexes in Python are always numbered from 0, so 0 is always the
first item in a list. In the steps just completed, you
indexed
into the list.


















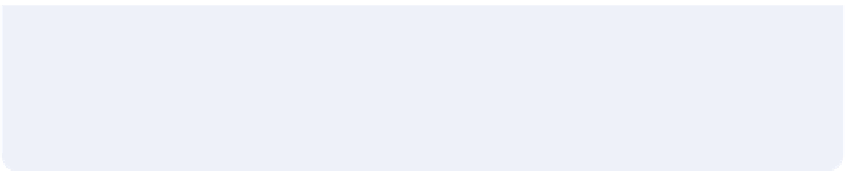
















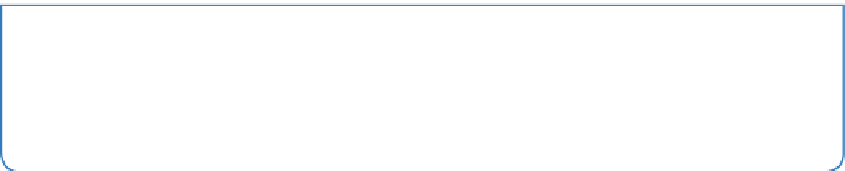