Java Reference
In-Depth Information
12.
The class
ResizableArrayBag
has an array that can grow in size as objects are added to the bag. Revise the class
so that its array also can shrink in size as objects are removed from the bag. Accomplishing this task will require
two new private methods, as follows:
●
The first new method checks whether we should reduce the size of the array:
private boolean
isTooBig()
This method returns true if the number of entries in the bag is less than half the size of the array and the
size of the array is greater than 20.
●
The second new method creates a new array that is three quarters the size of the current array and
then copies the objects in the bag to the new array:
private void
reduceArray()
Implement each of these two methods, and then use them in the definitions of the two
remove
methods.
13.
Consider the two private methods described in the previous exercise.
a.
The method
isTooBig
requires the size of the array to be greater than 20. What problem could occur if this
requirement is dropped?
b.
The method
reduceArray
is not analogous to the method
ensureCapacity
in that it does not reduce the
size of the array by one half. What problem could occur if the size of the array is reduced by one half
instead of three quarters?
14.
Define the method
union
, as described in Exercise 5 of the previous chapter, for the class
ResizableArrayBag
.
15.
Define the method
intersection
, as described in Exercise 6 of the previous chapter, for the class
ResizableArrayBag
.
16.
Define the method
difference
, as described in Exercise 7 of the previous chapter, for the class
ResizableArrayBag
.
P
ROJECTS
1.
Define a class
ArraySet
that represents a set and implements the interface described in Project 1a of the previous
chapter. Use the class
ResizableArrayBag
in your implementation. Then write a program that adequately demon-
strates your implementation.
2.
Repeat the previous project, but use a resizable array instead of the class
ResizableArrayBag
.
3.
Define a class
PileOfBooks
that implements the interface described in Project 2 of the previous chapter. Use a
resizable array in your implementation. Then write a program that adequately demonstrates your implementation.
4.
Define a class
Ring
that represents a ring and implements the interface described in Project 3 of the previous
chapter. Use a resizable array in your implementation. Then write a program that adequately demonstrates your
implementation.
5.
You can use either a set or a bag to create a spell checker. The set or bag serves as a dictionary and contains a col-
lection of correctly spelled words. To see whether a word is spelled correctly, you see whether it is contained in
the dictionary. Use this scheme to create a spell checker for the words in an external file. To simplify your task,
restrict your dictionary to a manageable size.
6.
Repeat the previous project to create a spell checker, but instead place the words whose spelling you want to
check into a bag. The difference between the dictionary (the set or bag containing the correctly spelled words) and
the bag of words to be checked is a bag of incorrectly spelled words.
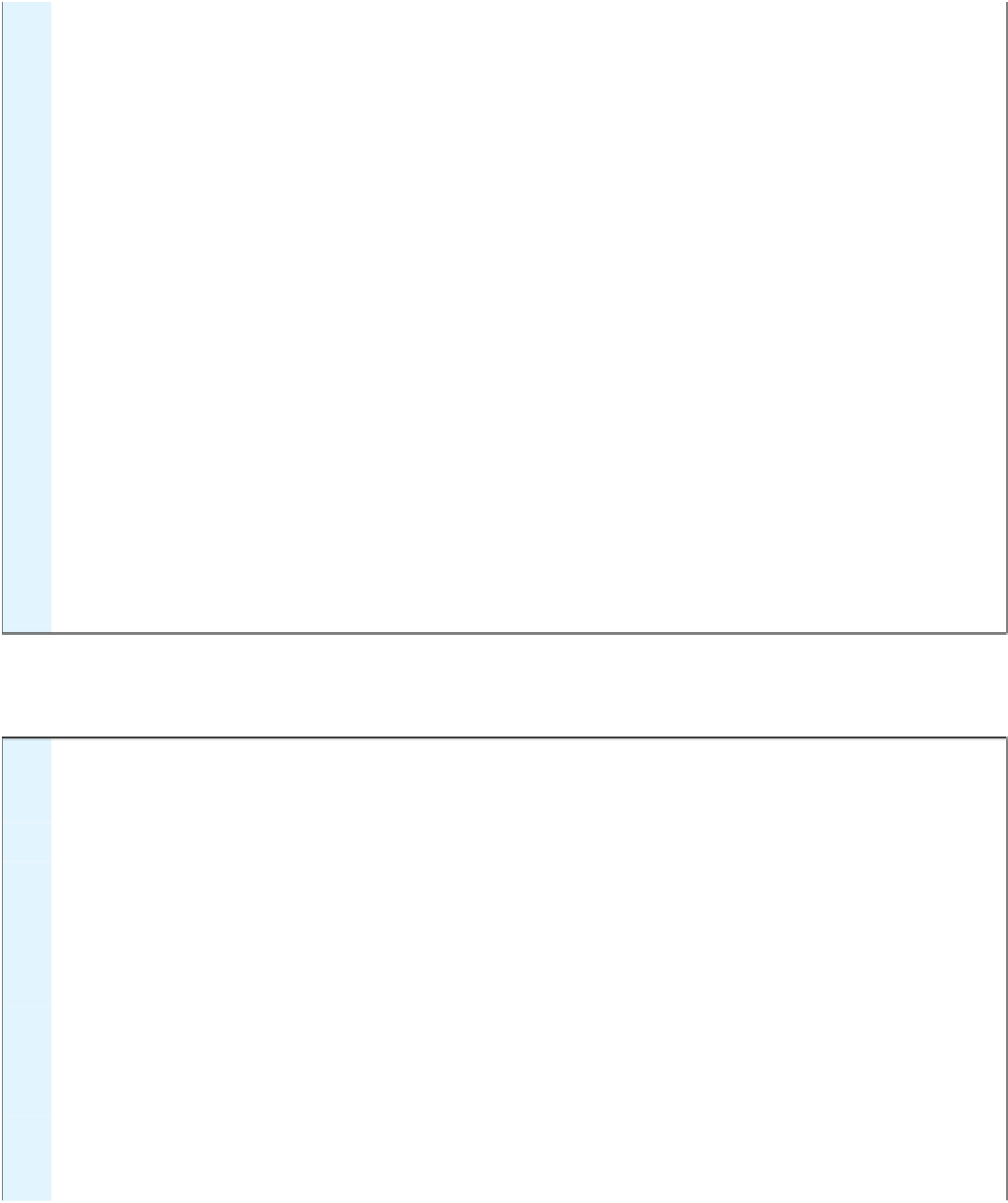