Java Reference
In-Depth Information
try
{
theCopy = (AList<T>)
super
.clone();
}
catch
(CloneNotSupportedException e)
{
throw new
Error(e.toString());
}
theCopy.list = (T[])list.clone();
for
(
int
index = 0; index < numberOfEntries; index++)
theCopy.list[index] = (T)list[index].clone();
return
theCopy;
}
// end clone
Note:
To make a deep clone of an array
a
of cloneable objects, you invoke
a.clone()
and
then clone each object in the array. For example, if
myArray
is an array of
Thing
objects, and
Thing
implements
Cloneable
, you would write
Thing[] clonedArray = (Thing[])myArray.clone();
for
(
int
index = 0; index < myArray.length; index++)
clonedArray[index] = (Thing)myArray[index].clone();
30.24
Now suppose that we want to add a
clone
method to a linked implementation of the ADT list, such as
the class
LList
of Chapter 14 or the class
LinkedChainBase
of Chapter 17. (The
clone
methods for
these classes are virtually identical.) Given the interface
Copyable
, we can begin the class in one of
the ways given in Segment 30.20. Ultimately, the class and the objects in the list must implement the
interface
Cloneable
. Thus,
LList
, for example, could begin as follows:
public class
LList<T
extends
Copyable>
implements
CloneableListInterface<T>
{
private
Node firstNode;
// reference to first node
private int
numberOfEntries;
. . .
The first part of the
clone
method would be like the code that you saw in Segment 30.22,
except that we would replace
AList
with
LList
. If we invoked only
super.clone()
, our method
would produce a shallow copy of the list, as Figure 30-9 illustrates. In other words, both the origi-
nal list and its clone would reference the same chain of nodes, and these nodes would reference one
set of data.
As before,
clone
needs to do more to perform a deep copy. It needs to clone the chain of nodes
as well as the data that the nodes reference. Figure 30-10 shows a list with its deep clone.
30.25
Cloning a node.
To clone the nodes in the chain, we need to add a method
clone
to the inner class
Node
. First, we add
implements
Cloneable
to the declaration of the class
Node
. Note that
Node
is
private in
LList
but protected in
LinkedChainBase
.
Node
's
clone
method begins like other
clone
methods, but then it goes on to clone the data portion of the node. We do not bother cloning the
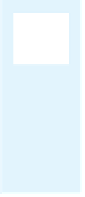

