Java Reference
In-Depth Information
Design Decision:
Should the method
toArray
return the array
bag
instead of a copy?
Suppose that we define
toArray
as follows:
public
String[] toArray()
{
return
bag;
}
// end toArray
This simple definition would certainly return an array of the bag's contents to a client. For example, the
statement
String[] bagArray = myBag.toArray();
provides a reference to an array of the entries in
myBag
. A client could use the variable
bagArray
to
display the contents of
myBag
.
The reference
bagArray
, however, is to the array
bag
itself. That is,
bagArray
is an alias for the
private instance variable
bag
within the object
myBag
, and therefore it gives the client direct access
to this private data. Thus, a client could change the contents of the bag without calling the class's
public methods. For instance, if
myBag
is the full bag pictured in Figure 2-3, the statement
bagArray[2] =
null
;
would change the entry Ted to
null
. Although this approach might sound good to you if the
intent is to remove Ted from the bag, doing so would destroy the integrity of the bag. In particu-
lar, the entries in the array
bag
would no longer be consecutive, and the count of the number of
entries in the bag would be incorrect.
Programming Tip
A class should not return a reference to an array that is a private data field.
Note:
A variable whose declared data type is
Object
can reference an object of any data
type. A collection whose entries are referenced by variables of type
Object
can contain
objects of various unrelated classes. In contrast, a variable having a generic data type can ref-
erence only an object of specific data types. A collection whose entries are referenced by
variables of a generic type can contain only objects of classes related by inheritance. Gener-
ics enable you to restrict the data types of the entries in your collections.
Question 4
In the previous method
toArray
, does the value of
numberOfEntries
equal
bag.length
in general?
Question 5
Suppose that the previous method
toArray
gave the new array
result
the same
length as the array
bag
. How would a client get the number of entries in the returned array?
Question 6
Suppose that the previous method
toArray
returned the array
bag
instead of
returning a new array such as
result
. If
myBag
is a bag of five entries, what effect would the
following statements have on the array
bag
and the field
numberOfEntries
?
Object[] bagArray = myBag.toArray();
bagArray[0] =
null
;
Question 7
The body of the method
toArray
could consist of one
return
statement if you
call the method
Arrays.copyOf
. Make this change to
toArray
.
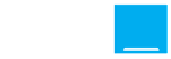



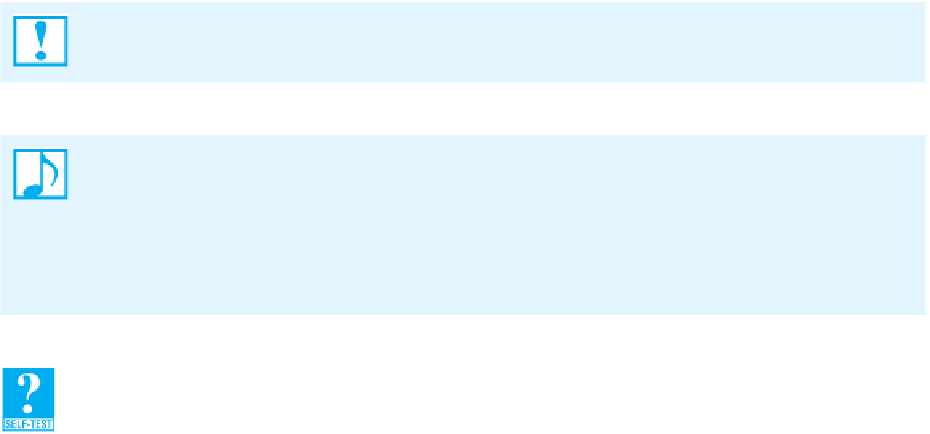

