Java Reference
In-Depth Information
With this core, we will be able to construct a bag, add objects to it, and look at the result. We will
not implement the remaining methods until these core methods work correctly.
Note:
Methods such as
add
and
remove
that can alter the underlying structure of a collec-
tion are likely to have the most involved implementations. In general, you should define such
methods before the others in the class. But since we can't test
remove
before
add
is correct,
we will delay implementing it until after
add
is completed and thoroughly tested.
Programming Tip:
When defining a class, implement and test a group of core methods.
Begin with methods that add to a collection of objects and/or have involved implementations.
The data fields.
Before we define any of the core methods, we need to consider the class's data
fields. Since the bag will hold a group of objects, one field can be an array of these objects. The
length of the array defines the bag's capacity. We can let the client specify this capacity, and we can
also provide a default capacity. In addition, we will want to track the current number of entries in
the bag. Thus, we can define the following data fields for our class,
private final
T[] bag;
private static final int
DEFAULT_CAPACITY = 25;
private int
numberOfEntries;
and add them to our earlier UML representation of the class in Figure 1-2 of the previous chapter.
The resulting notation appears in Figure 2-2.
FIGURE 2-2
UML notation for the class
ArrayBag
, including the class's data fields
ArrayBag
-bag: T[]
-DEFAULT_CAPACITY: integer
-numberOfEntries: integer
+getCurrentSize(): integer
+isFull(): boolean
+isEmpty(): boolean
+add(newEntry: T): boolean
+remove(): T
+remove(anEntry: T): boolean
+clear(): void
+getFrequencyOf(anEntry: T): integer
+contains(anEntry: T): boolean
+toArray(): T[]
2.7
About the constructors.
A constructor for this class must create the array
bag
. Notice that the dec-
laration of the data field
bag
in the previous segment does not create an array. Forgetting to create
an array in a constructor is a common mistake. To create the array, the constructor must specify the
array's length, which is the bag's capacity. And since we are creating an empty bag, the constructor
should also initialize the field
numberOfEntries
to zero.



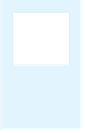

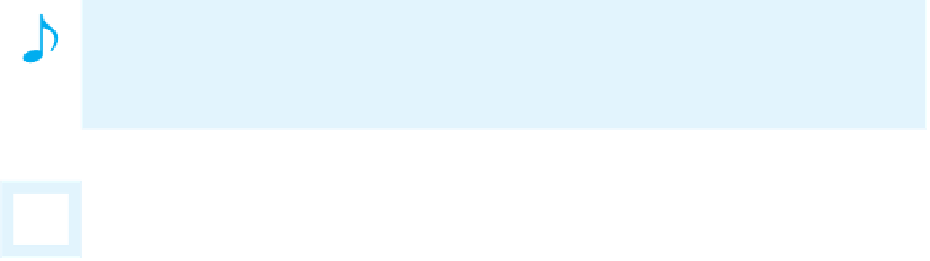

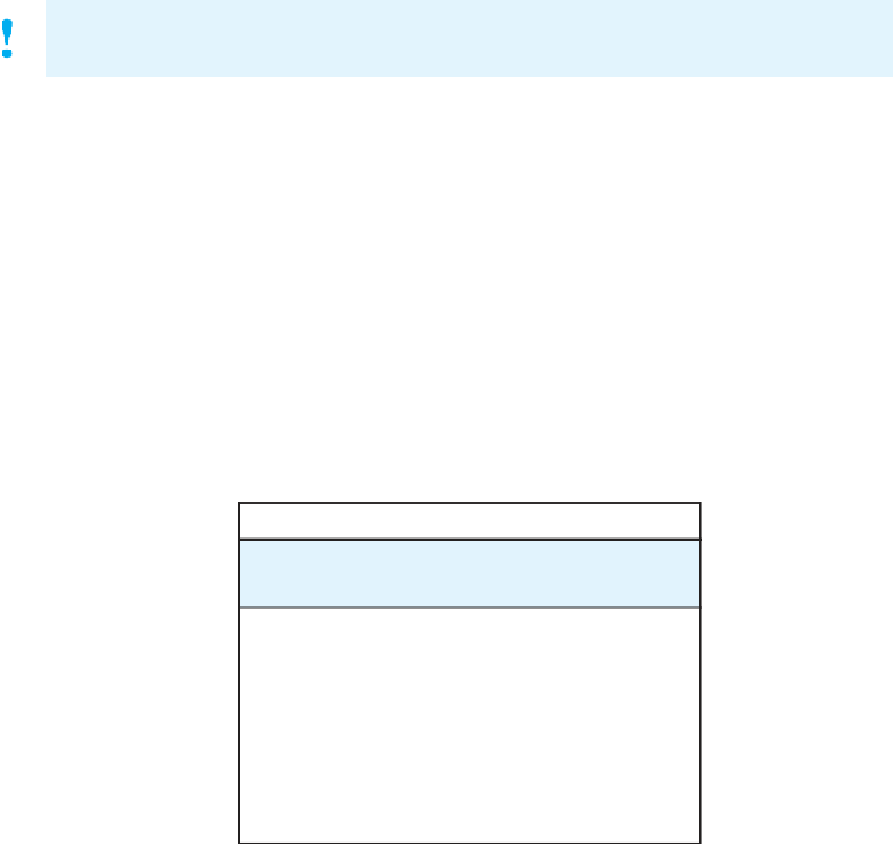