Java Reference
In-Depth Information
Ignore the invalid situations. A method could simply do nothing when given invalid
data. Doing absolutely nothing, however, leaves the client without knowledge of what
happened.
●
Guess at the client's intention. Like the previous option, this choice can cause problems
for the client.
●
Return a value that signals a problem. For example, if a client tries to remove an entry
from an empty bag, the
remove
method could return
null
. The value returned must be
something that cannot be in the bag.
●
Return a boolean value that indicates the success or failure of an operation.
●
Throw an exception.
●
Note:
Throwing an exception is often a desirable way for a Java method to react to unusual
events that occur during its execution. The method can simply report a problem without
deciding what to do about it. The exception enables each client to do what is needed in its
own particular situation. However, the method invocation in the client must appear within a
try
block. For simplicity right now, we will adopt the philosophy that methods should throw
exceptions only in truly exceptional circumstances, when no other reasonable solution exists.
Note:
A first draft of an ADT's specifications often overlooks or ignores situations that you
really need to consider. You might intentionally make these omissions to simplify this first
draft. Once you have written the major portions of the specifications, you can concentrate on
the details that make the specifications complete.
1.13
As your specifications become more detailed, they increasingly should reflect your choice of pro-
gramming language. Ultimately, you can write Java headers for the bag's methods and organize them
into a Java interface for the class that will implement the ADT. The Java interface in Listing 1-1 con-
tains the methods for an ADT bag and detailed comments that describe their behaviors. Recall that a
class interface does not include data fields, constructors, private methods, or protected methods.
For now, the items in the bag will be objects of the same class. For example, we could have a
bag of strings. To accommodate entries of any one class type, the bag methods use a generic type
T
for each entry. To give meaning to the identifier
T
, we must write
<T>
after the name of the inter-
face. Once the actual data type is chosen within a client, the compiler will use that data type wher-
ever
T
appears.
As you examine the interface, notice the decisions that were made to address the unusual situations
mentioned in the previous segment. In particular, each of the methods
add
,
remove
, and
contains
returns a value. Since our programming language is Java, notice that one of the
remove
methods returns
a reference to an entry, not the entry itself.
Although writing an interface before implementing a class is certainly not required, doing so
enables you to document your specifications in a concise way. You then can use the code in the
interface as an outline for the actual class. Having an interface also provides a data type for a bag
that is independent of a particular class definition. The next two chapters will develop different




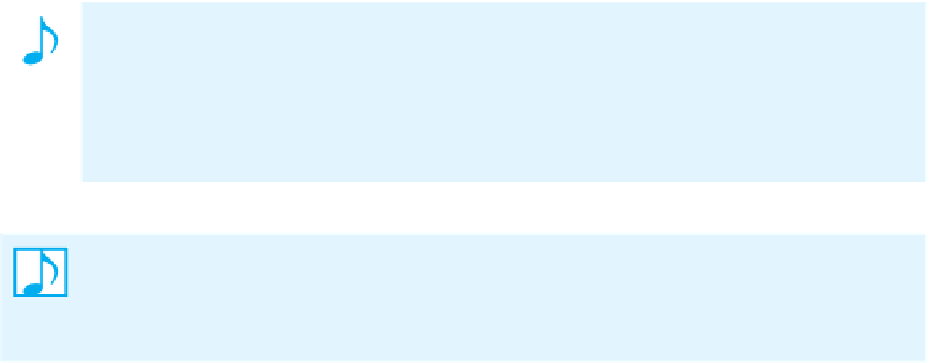