Java Reference
In-Depth Information
C
HAPTER
S
UMMARY
The interface
Iterator
specifies three methods:
hasNext
,
next
, and
remove
. An iterator that implements
this interface need not provide a
remove
operation. Instead, the method
remove
would throw the exception
UnsupportedOperationException
.
●
The interface
ListIterator
specifies nine methods, including the three methods that
Iterator
specifies. They are
hasNext
,
next
,
hasPrevious
,
previous
,
nextIndex
,
previousIndex
,
add
,
remove
, and
set
. The methods
add
,
remove
, and
set
are optional in the sense that they can throw the exception
UnsupportedOperationException
instead of affecting the list.
●
You can implement each of the interfaces
Iterator
and
ListIterator
as its own class. This class could be an
inner class of the class that implements the ADT in question, or it could be public and separate from the ADT's class.
●
An inner class iterator enables you to have several independent iterators that traverse a collection. It also
allows the iterator direct access to the underlying data structure, so its implementation can be efficient.
●
A separate class iterator also allows multiple and distinct iterations to exist simultaneously. However, since the
iterator can access the list's data fields only indirectly via ADT operations, the iteration takes more time than
one performed by an inner class iterator. On the other hand, the implementation is usually straightforward.
●
Certain ADTs do not provide sufficient public access to their data to make a separate class iterator possible.
However, to provide an iterator for an ADT's implementation that exists and cannot be altered, you might
need to define a separate class iterator.
●
P
ROGRAMMING
T
IPS
All of the exceptions mentioned in the interfaces
Iterator
and
ListIterator
are run-time exceptions, so no
throws
clause is necessary in any of the methods' headers. In addition, you do not have to write
try
and
catch
blocks when you invoke these methods. However, you will need to import
NoSuchElementException
from the
package
java.util
. The other exceptions are in
java.lang
, so no
import
statement is necessary for them.
●
A class that defines an inner class iterator should implement the interface
Iterable
. A client of the class can
then use a for-each loop to traverse the objects in an instance of the class.
●
E
XERCISES
1.
Suppose that
nameList
is a list that contains the following strings:
Kyle, Cathy, Sam, Austin, Sara.
What output is
produced by the following sequence of statements?
Iterator<String> nameIterator = nameList.getIterator();
System.out.println(nameIterator.next());
nameIterator.next();
System.out.println(nameIterator.next());
nameIterator.remove();
System.out.println(nameIterator.next());
displayList(nameList);
2.
Repeat Exercise 1, but instead use the following statements:
Iterator<String> nameIterator = nameList.getIterator();
nameIterator.next();
nameIterator.remove();
nameIterator.next();
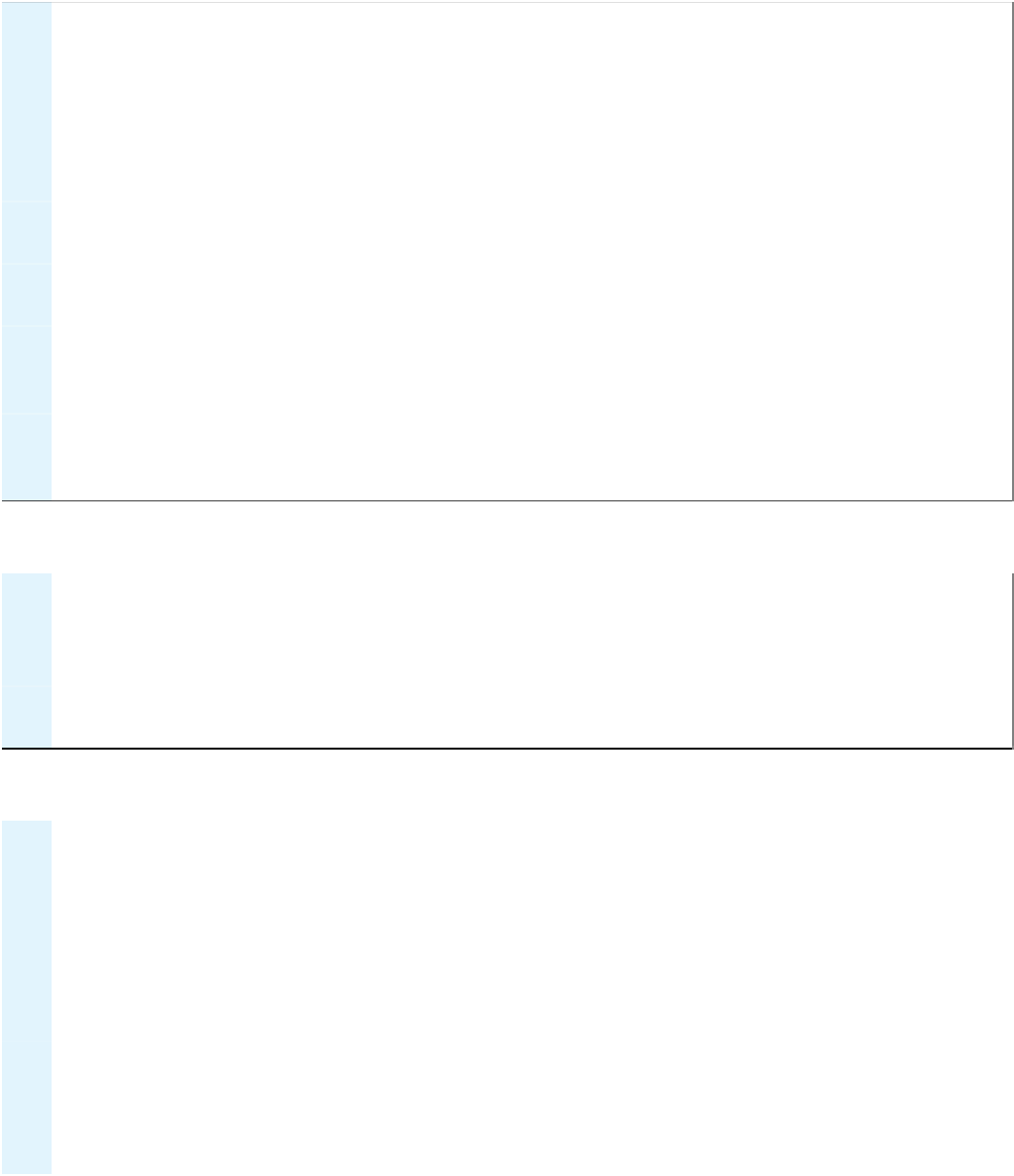
