Java Reference
In-Depth Information
nextIndex++;
// advance iterator
return
nextEntry;
}
else
throw new
NoSuchElementException("Illegal call to next(); " +
"iterator is after end of list.");
}
// end next
15.28
The
remove
method
. Removing an entry from the list involves shifting entries within the array
list
. Since we have already developed that code for the list's
remove
method, we will call it
instead of accessing the array
list
directly. To do that, we need the position number of the list
entry to be removed, rather than its array index. Recall from Segment 12.1 that the position number
of an entry in a list begins at 1, so it is 1 larger than the corresponding array index.
Figure 15-8 illustrates how to use
nextIndex
in this implementation. The figure shows the
array of list entries and the index
nextIndex
just before the call to
next
, just after the call to
next
but before the call to
remove
, and just after the call to
remove
. Part
b
shows that
next
returns a ref-
erence to the next entry,
Chris
, in the iteration and then increments
nextIndex
. The method
remove
must remove this entry from the list. Since
nextIndex
is now 1 larger than the index of
Chris
, it is the position number of the list entry that must be removed. After
Chris
is removed in
Part
c
, the next entry—
Deb
—moves to the next lower-numbered position in the array. Thus,
remove
decrements
nextIndex
so that it remains the index of the next entry in the iteration.
FIGURE 15-8
The array of list entries and
nextIndex
(a) just before the call to
next
; (b) just after the call to
next
but before the call to
remove
;
(c) after the call to
remove
(c) After
remove()
removes
Chris
(a) Before
next()
(b) After
next()
returns
Chris
Art
Art
Art
Bart
Bart
Bart
Iterator cursor
nextIndex
= 2
Iterator cursor
nextIndex
= 2
Chris
Chris
Deb
Iterator cursor
nextIndex
= 3
Deb
Deb
Elly
Elly
Elly
The method
remove
has the following implementation within the inner class
IteratorForArrayList
:
public void
remove()
{
if
(wasNextCalled)
{
// nextIndex was incremented by the call to next, so it
// is the position number of the entry to be removed
ArrayListWithIterator.
this
.remove(nextIndex);
nextIndex--;
// index of next entry in iteration




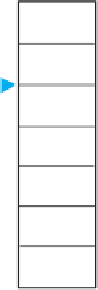

