Java Reference
In-Depth Information
13.10
The method
remove
.
Removing a list entry at an arbitrary position is like a student leaving room
A in our example in Segment 13.3. We need to shift existing entries to avoid a gap in the array,
except when removing the list's last entry. The following implementation uses a private method
removeGap
to handle the details of moving data within the array. Like the method
add
,
remove
is
responsible for checking the validity of the given position. Note how this check also ensures that
the list is not empty.
public
T remove(
int
givenPosition)
{
VideoNote
Completing the class
AList
T result =
null
;
// return value
if
((givenPosition >= 1) && (givenPosition <= numberOfEntries))
{
assert
!isEmpty();
result = list[givenPosition - 1];
// get entry to be removed
// move subsequent entries toward entry to be removed,
// unless it is last in list
if
(givenPosition < numberOfEntries)
removeGap(givenPosition);
numberOfEntries--;
}
// end if
return
result;
// return reference to removed entry, or
// null if either list is empty or givenPosition
// is invalid
}
// end remove
Question 7
Since the method
remove
does not explicitly check for an empty list, why is
the assertion given in the method true?
Question 8
When a list is empty, how does
remove
return
null
?
13.11
The private method
removeGap
.
The following private method
removeGap
shifts list entries within
the array, as Figure 13-5 illustrates. Beginning with the entry after the one to be removed and con-
tinuing until the end of the list,
removeGap
moves each entry to its next lower position.
// Shifts entries that are beyond the entry to be removed to the
// next lower position.
// Precondition: 1 <= givenPosition < numberOfEntries;
// numberOfEntries is list's length before removal.
private void
removeGap(
int
givenPosition)
{
assert
(givenPosition >= 1) && (givenPosition < numberOfEntries);
int
removedIndex = givenPosition - 1;
int
lastIndex = numberOfEntries - 1;
for
(
int
index = removedIndex; index < lastIndex; index++)
list[index] = list[index + 1];
}
// end removeGap
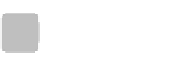











