Java Reference
In-Depth Information
10.15
Example.
When typing at your keyboard, you might make a mistake. If you backspace to correct
your mistake, what logic is used to decipher your intention? For example, if the symbol
←
repre-
sents a backspace, and you type
cm
←
ompte
←←
utr
←
er
the result should be
computer
Each backspace erases the previous character entered.
To replicate this process, as characters are entered, we retain them in an ADT. We want this
ADT to be stacklike so we can access the most recently entered character. But since we ultimately
want the corrected characters to be in the order in which they were entered, we want the ADT to
also behave like a queue. The ADT deque can satisfy these requirements.
The following pseudocode uses a deque to read and display a line of keyboard input:
//
read a line
d =
a new empty dequeue
while
(
not end of line
)
{
character =
next character read
if
(character ==
←
)
d.removeBack()
else
d.addToBack(character)
}
//
display the corrected line
while
(!d.isEmpty())
System.out.print(d.removeFront())
System.out.println()
When we concluded the capital gain example, Segment 10.12 noted that our queue contained
individual shares of stock. Since a typical stock transaction involves more than one share, repre-
senting a transaction as one object is more natural. But you saw that the transaction object must
have set methods if we use a queue. That would not be the case if we used a deque instead.
10.16
In this section, we revise the implementation, but not the design, of the class
StockLedger
that was intro-
duced in Segment 10.10. We also revise the class
StockPurchase
so that it represents the purchase of
n
shares of stock at
d
dollars per share, as Segment 10.12 suggests. The revised class has the data fields
shares
and
cost
, a constructor, and the accessor methods
getNumberOfShares
and
getCostPerShare
.
We can revise the implementation of the class
StockLedger
given in Segment 10.11 as fol-
lows. The data field
ledger
is now an instance of a deque instead of a queue. The method
buy
cre-
ates an instance of
StockPurchase
and places it at the back of the deque, as follows:
public void
buy(
int
sharesBought,
double
pricePerShare)
{
StockPurchase purchase =
new
StockPurchase(sharesBought, pricePerShare);
ledger.addToBack(purchase);
}
// end buy
The method
sell
is more involved. It must remove a
StockPurchase
object from the front of the
deque and decide whether that object represents more shares than the number sold. If it does, the
method creates a new instance of
StockPurchase
to represent the shares that remain in the portfolio.
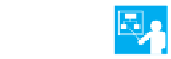
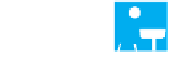





