Java Reference
In-Depth Information
myBag.add("40");
myBag.add("50");
myBag.add("10");
myBag.add("60");
myBag.add("20");
int
numberOfEntries = myBag.getCurrentSize();
String[] entries = myBag.toArray();
for
(
int
index = 0; index < numberOfEntries; index++)
System.out.print(entries[index] + " ");
b.
What methods, if any, in
LinkedBag
could be affected by the change to the method
add
when they
execute? Why?
3.
Repeat Exercise 2 in the previous chapter for the class
LinkedBag
.
4.
Revise the definition of the method
remove
, as given in Segment 3.21, so that it removes a random entry
from a bag. Would this change affect any other method within the class
LinkedBag
?
5.
Define a method
removeEvery
for the class
LinkedBag
that removes all occurrences of a given entry from a bag.
6.
Suppose that a bag contains
Comparable
objects. Define the following methods for the class
LinkedBag
:
•
The method
getMin
that returns the smallest object in a bag
•
The method
getMax
that returns the largest object in a bag
7.
Repeat Exercise 10 in the previous chapter for the class
LinkedBag
.
8.
Define an
equals
method for the class
LinkedBag
. Consult Exercise 11 in the previous chapter for details about
this method.
9.
Define the method
union
, as described in Exercise 5 of Chapter 1, for the class
LinkedBag
.
10.
Define the method
intersection
, as described in Exercise 6 of Chapter 1, for the class
LinkedBag
.
11.
Define the method
difference
, as described in Exercise 7 of Chapter 1, for the class
LinkedBag
.
12.
In a
doubly linked chain
, each node can reference the previous node as well as the next node. Figure 3-11 shows
a doubly linked chain and its head reference. Define a class to represent a node in a doubly linked chain. Write the
class as an inner class of a class that implements the ADT bag. You can omit set and get methods.
FIGURE 3-11
A doubly linked chain for Exercises 12, 13, 14, and 15, and Project 7
firstNode
13.
Repeat Exercise 12, but instead write the class within a package that contains an implementation of the ADT bag.
Set and get methods will be necessary.
14.
List the steps necessary to add a node to the beginning of the doubly linked chain shown in Figure 3-11.
15.
List the steps necessary to remove the first node from the beginning of the doubly linked chain shown in Figure 3-11.
P
ROJECTS
1.
Write a program that thoroughly tests the class
LinkedBag
.
2.
Listing 3-4 shows the inner class
Node
with set and get methods. Revise the class
LinkedBag
so that it invokes
these set and get methods instead of accessing the private data fields
data
and
next
directly by name.




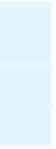





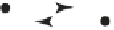
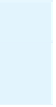












