Java Reference
In-Depth Information
for (var bottles = 10 ; bottles > 0 ; bottles--) {
alert("There were " + bottles + " green bottles, hanging
on the
↵
wall. And if one green bottle should accidently fall,
there'd be
↵
" + (bottles-1) + " green bottles hanging on the wall");
}
Each of the three parts are optional, and the code could be written as:
var bottles = 10; // bottles is initialized here instead
for ( ; bottles > 0 ; ) { // empty initialization and
increment
alert("There were " + bottles + " green bottles, hanging
on the
↵
wall. And if one green bottle should accidently fall,
there'd be
↵
" + (bottles-1) + " green bottles hanging on the wall");
bottles--; // increment moved into code block
}
As you can see, it's possible to use a
while
loop, a
do ... while
loop, or a
for
loop
to achieve the same results. A
for
loop is the most common as it keeps all the details of
the loop (the initialization, condition, and increment) in one place and separate from the
code block.
Nested
for
Loops
You can place a loop inside another loop to create a nested loop. It will have an inner loop
that will run all the way through before the next step of the outer loop occurs.
Here's an example that produces a multiplication table up to 12 x 12:
for(var n=1 ; n<13 ; n++){
for(var m=1 ; m<13 ; m++){
console.log(m + " multiplied by " + n + " is " + n*m);
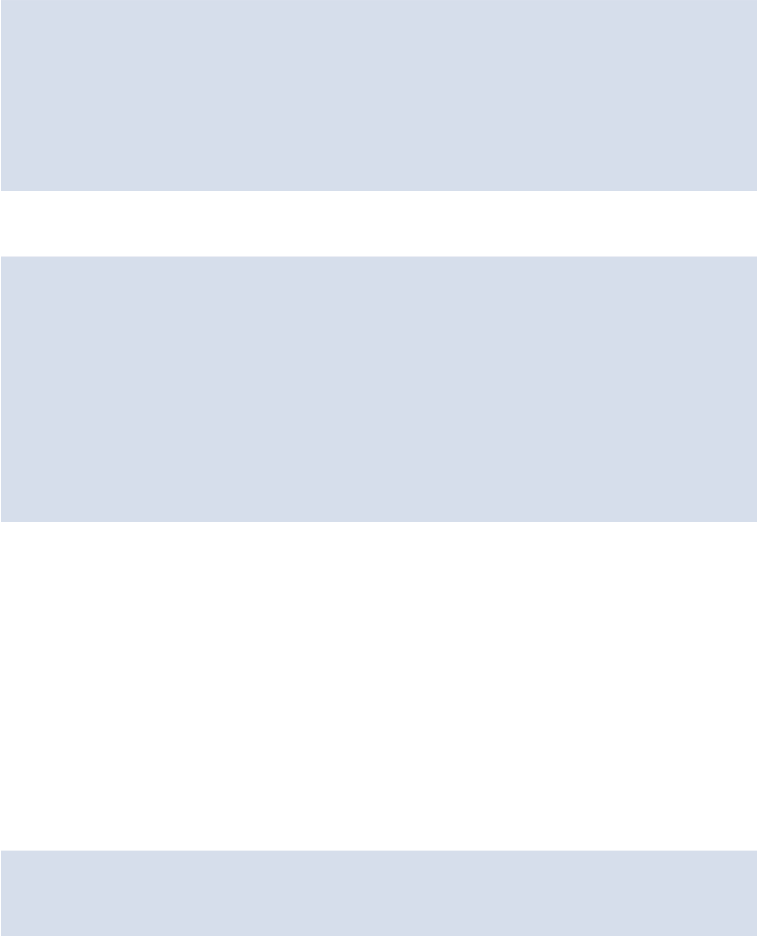