Game Development Reference
In-Depth Information
1. using UnityEngine;
2. using System.Collections; 3.
4. public class BulletHoleMaker : MonoBehaviour { 5.
6.
//Object to instantiate as hole
7.
public GameObject holePrefab;
8.
9.
//Seconds to wait before destroying hole object
10.
public float holeLife = 15;
11.
12.
void Start () { 13.
14.
}
15.
16.
void Update () { 17.
18.
}
19.
20.
//Receive OnRaycastHit message and generate hole at hit position
21.
void OnRaycastHit(RaycastHit hit){
22.
GameObject hole = (GameObject) Instantiate(holePrefab);
23.
hole.transform.position = hit.point;
24.
//If there is a rigid body, add the hole as a child to it
25.
//This makes the hole move along with the hit object
26.
if(hit.rigidbody != null){
27.
hole.transform.parent = hit.transform;
28.
}
29.
//Since we use a quad, we rotate it towards inside
30.
//This might be different if you use another shape
31.
hole.transform.LookAt(hit.point - hit.normal);
32.
//move it away from hit surface with a tiny amount
33.
//This ensures that the hole is always on top
34.
hole.transform.Translate(0, 0, -0.0125f);
35.
36.
//Invoke destruction after a while













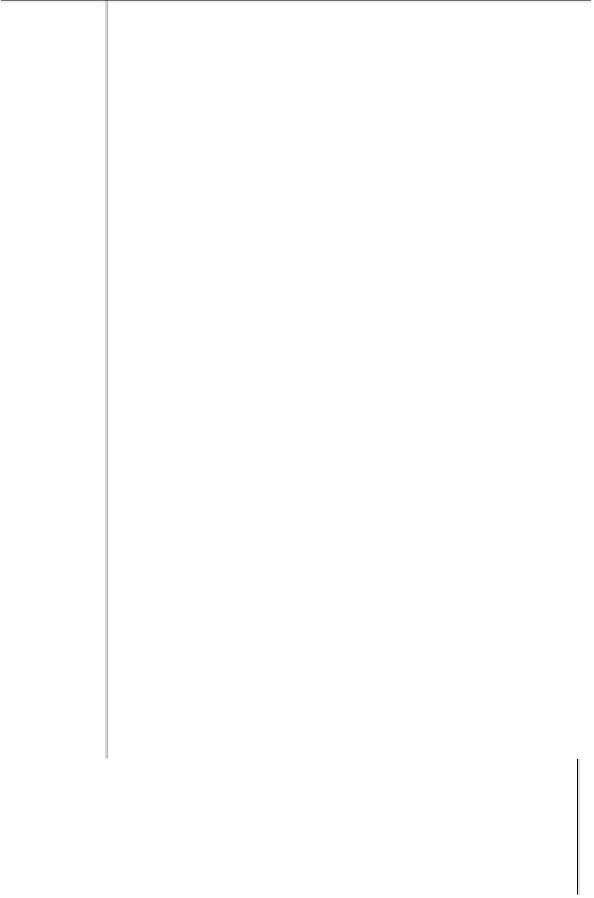