Java Reference
In-Depth Information
Algebraic
operator
Java equality or
relational operator
Sample Java
condition
Meaning of Java condition
Relational operators
>
>
x > y
x
is greater than
y
<
<
x < y
x
is less than
y
≥
>=
x >= y
x
is greater than or equal to
y
≤
<=
x <= y
x
is less than or equal to
y
Fig. 2.14
|
Equality and relational operators. (Part 2 of 2.)
Figure 2.15 uses six
if
statements to compare two integers input by the user. If the
condition in any of these
if
statements is
true
, the statement associated with that
if
state-
ment executes; otherwise, the statement is skipped. We use a
Scanner
to input the integers
from the user and store them in variables
number1
and
number2
. The program
compares
the numbers and displays the results of the comparisons that are true.
1
// Fig. 2.15: Comparison.java
2
// Compare integers using if statements, relational operators
3
// and equality operators.
4
import
java.util.Scanner;
// program uses class Scanner
5
6
public class
Comparison
7
{
8
// main method begins execution of Java application
9
public static void
main(String[] args)
10
{
11
// create Scanner to obtain input from command line
12
Scanner input =
new
Scanner(System.in);
13
14
int
number1;
// first number to compare
15
int
number2;
// second number to compare
16
17
System.out.print(
"Enter first integer: "
);
// prompt
18
number1 = input.nextInt();
// read first number from user
19
20
System.out.print(
"Enter second integer: "
);
// prompt
21
number2 = input.nextInt();
// read second number from user
22
23
if
(number1 == number2)
System.out.printf(
"%d == %d%n"
, number1, number2);
24
25
26
if
(number1 != number2)
System.out.printf(
"%d != %d%n"
, number1, number2);
27
28
29
if
(number1 < number2)
System.out.printf(
"%d < %d%n"
, number1, number2);
30
Fig. 2.15
|
Compare integers using
if
statements, relational operators and equality operators.
(Part 1 of 2.)

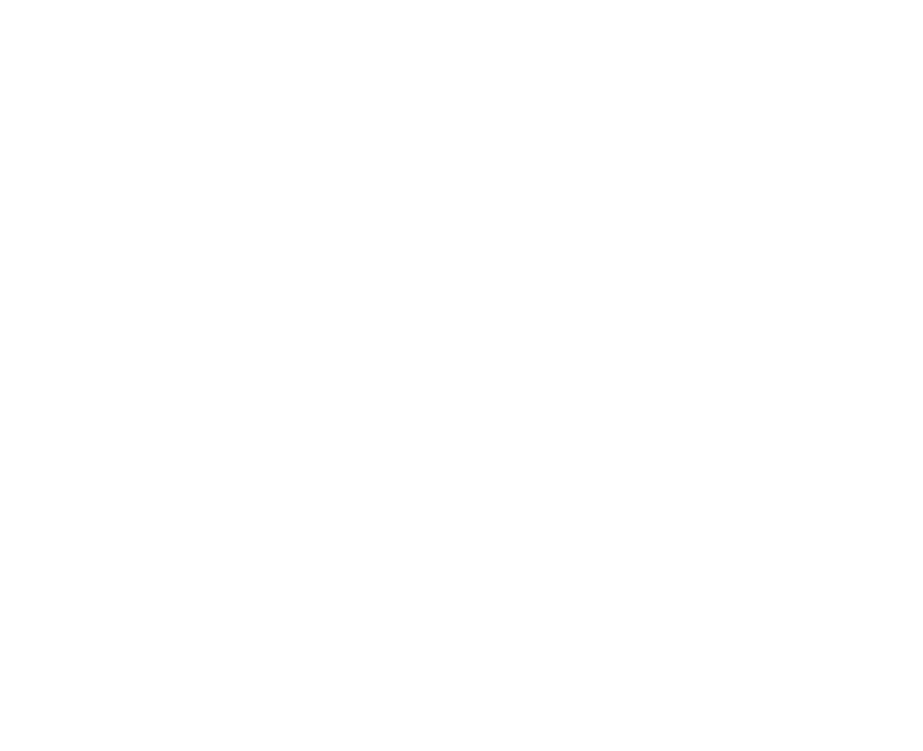




















