Java Reference
In-Depth Information
20
private final
UIManager.LookAndFeelInfo[] looks;
21
private final
String[] lookNames;
// look-and-feel names
22
private final
JRadioButton[] radio;
// for selecting look-and-feel
23
private final
ButtonGroup group;
// group for radio buttons
24
private final
JButton button;
// displays look of button
25
private final
JLabel label;
// displays look of label
26
private final
JComboBox<String> comboBox;
// displays look of combo box
27
28
// set up GUI
29
public
LookAndFeelFrame()
30
{
31
super
("Look and Feel Demo");
32
33
// get installed look-and-feel information
looks = UIManager.getInstalledLookAndFeels();
34
35
lookNames =
new
String[looks.length];
36
37
// get names of installed look-and-feels
38
for
(
int
i =
0
; i < looks.length; i++)
39
lookNames[i] = looks[i].getName();
40
41
JPanel northPanel =
new
JPanel();
42
northPanel.setLayout(
new
GridLayout(
3
,
1
,
0
,
5
));
43
44
label =
new
JLabel(
"This is a "
+ lookNames[
0
] +
" look-and-feel"
,
45
SwingConstants.CENTER
);
46
northPanel.add(label);
47
48
button =
new
JButton(
"JButton"
);
49
northPanel.add(button);
50
51
comboBox =
new
JComboBox<String>(lookNames);
52
northPanel.add(comboBox);
53
54
// create array for radio buttons
55
radio =
new
JRadioButton[looks.length];
56
57
JPanel southPanel =
new
JPanel();
58
59
// use a GridLayout with 3 buttons in each row
60
int
rows = (
int
) Math.ceil(radio.length /
3.0
);
61
southPanel.setLayout(new GridLayout(rows,
3
));
62
63
group =
new
ButtonGroup();
// button group for look-and-feels
64
ItemHandler handler =
new
ItemHandler();
// look-and-feel handler
65
66
for
(
int
count =
0
; count < radio.length; count++)
67
{
68
radio[count] =
new
JRadioButton(lookNames[count]);
69
radio[count].addItemListener(handler);
// add handler
70
group.add(radio[count]);
// add radio button to group
71
southPanel.add(radio[count]);
// add radio button to panel
72
}
Fig. 22.9
|
Look-and-feel of a Swing-based GUI. (Part 2 of 3.)
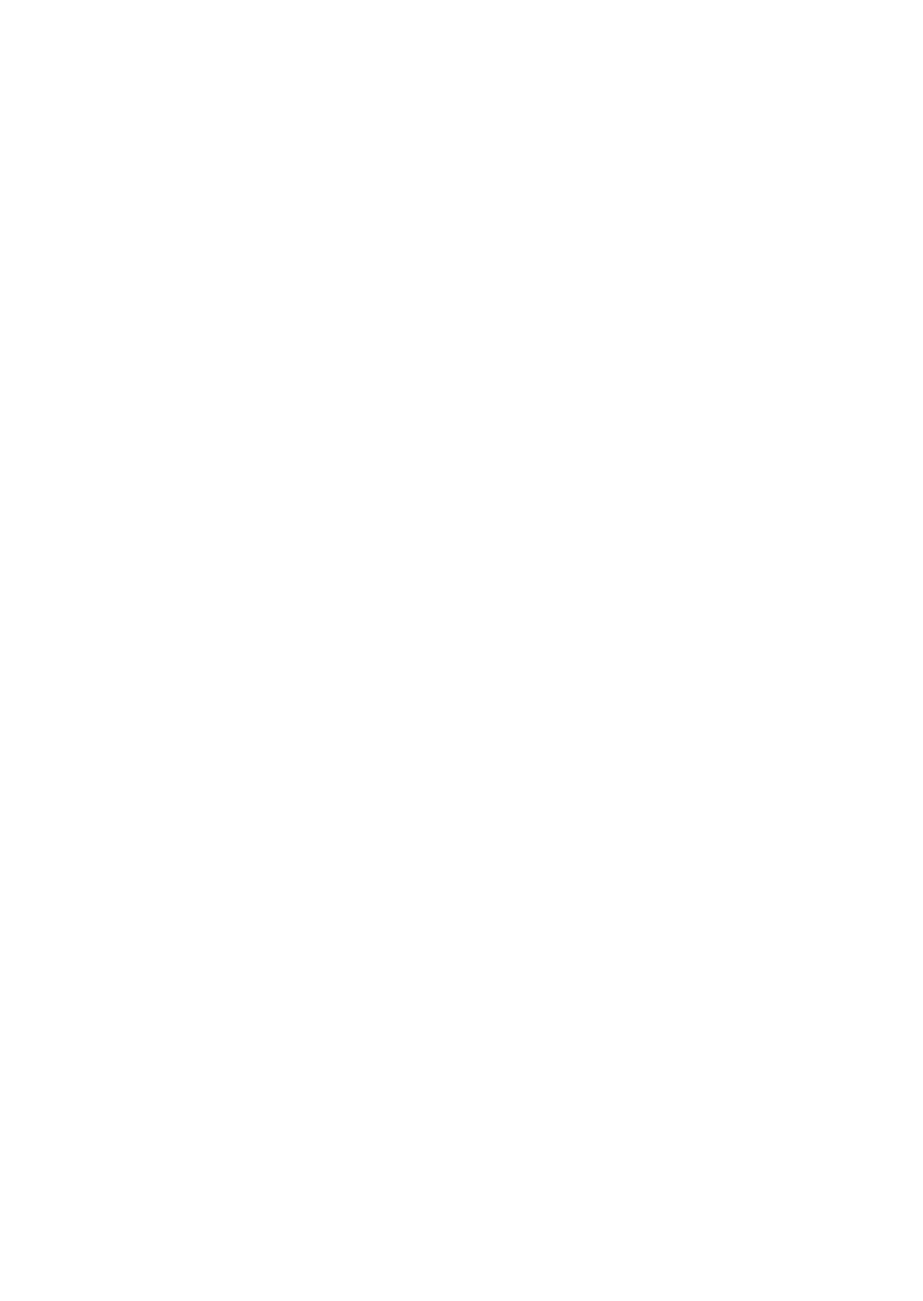



