Java Reference
In-Depth Information
18
stack.print();
stack.push(
5
);
stack.print();
19
20
21
22
// remove items from stack
23
try
24
{
25
int
removedItem;
26
27
while
(
true
)
28
{
29
removedItem = stack.pop();
// use pop method
30
System.out.printf(
"%n%d popped%n"
, removedItem);
31
stack.print();
32
}
33
}
34
catch
(EmptyListException emptyListException)
35
{
36
emptyListException.printStackTrace();
37
}
38
}
39
}
// end class StackInheritanceTest
The stack is: -1
The stack is: 0 -1
The stack is: 1 0 -1
The stack is: 5 1 0 -1
5 popped
The stack is: 1 0 -1
1 popped
The stack is: 0 -1
0 popped
The stack is: -1
-1 popped
Empty stack
com.deitel.datastructures.EmptyListException: stack is empty
at com.deitel.datastructures.List.removeFromFront(List.java:81)
at com.deitel.datastructures.StackInheritance.pop(
StackInheritance.java:22)
at StackInheritanceTest.main(StackInheritanceTest.java:29)
Fig. 21.11
|
Stack manipulation program. (Part 2 of 2.)
Forming a Stack Class Via Composition Rather Than Inheritance
A better way to implement a stack class is by reusing a list class through
composition
.
Figure 21.12 uses a
private
List<T>
(line 7) in class
StackComposition<T>
's declaration.
Composition enables us to hide the
List<T>
methods that should not be in our stack's
public
interface. We provide
public
interface methods that use only the required
List<T>
methods. Implementing each stack method as a call to a
List<T>
method is called
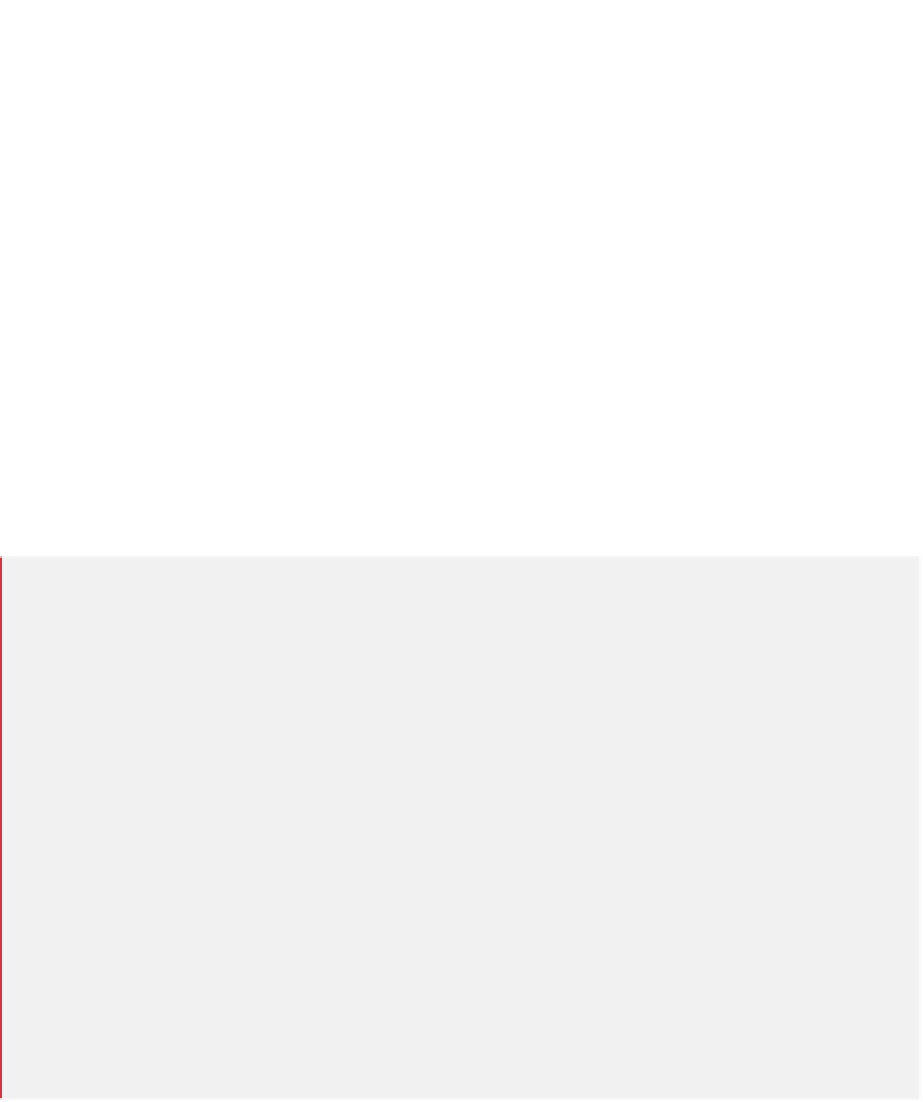








