Java Reference
In-Depth Information
5
public class
FactorialCalculator
6
{
7
// recursive method factorial (assumes its parameter is >= 0)
public static
BigInteger factorial(BigInteger number)
{
if
(number.compareTo(
BigInteger.ONE
) <=
0
)
// test base case
return
BigInteger.ONE
;
// base cases: 0! = 1 and 1! = 1
else
// recursion step
return
number.multiply(
factorial(number.subtract(
BigInteger.ONE
)));
}
8
9
10
11
12
13
14
15
16
17
// output factorials for values 0-50
18
public static void
main(String[] args)
19
{
20
// calculate the factorials of 0 through 50
21
for
(
int
counter =
0
; counter <=
50
; counter++)
22
System.out.printf(
"%d! = %d%n"
, counter,
23
factorial(BigInteger.valueOf(counter))
);
24
}
25
}
// end class FactorialCalculator
0! = 1
1! = 1
2! = 2
3! = 6
...
21! = 51090942171709440000
22! = 1124000727777607680000
...
47! = 258623241511168180642964355153611979969197632389120000000000
48! = 12413915592536072670862289047373375038521486354677760000000000
49! = 608281864034267560872252163321295376887552831379210240000000000
50! = 30414093201713378043612608166064768844377641568960512000000000000
Fig. 18.4
|
Factorial calculations with a recursive method. (Part 2 of 2.)
21! and larger values no longer cause overflow
Since
BigInteger
is
not
a primitive type, we can't use the arithmetic, relational and
equality operators with
BigInteger
s; instead, we must use
BigInteger
methods to
perform these tasks. Line 10 tests for the base case using
BigInteger
method
compareTo
.
This method compares the
BigInteger
number
that calls the method to the method's
BigInteger
argument. The method returns
-1
if the
BigInteger
that calls the method is
less than the argument,
0
if they're equal or
1
if the
BigInteger
that calls the method is
greater than the argument. Line 10 compares the
BigInteger
number
with the
BigInteger
constant
ONE
, which represents the integer value 1. If
compareTo
returns
-1
or
0
, then
number
is less than or equal to 1 (the base case) and the method returns the constant
BigInteger.ONE
. Otherwise, lines 13-14 perform the recursion step using
BigInteger
methods
multiply
and
subtract
to implement the calculations required to multiply
number
by the factorial of
number
-
1
. The program's output shows that
BigInteger
han-
dles the large values produced by the factorial calculation.
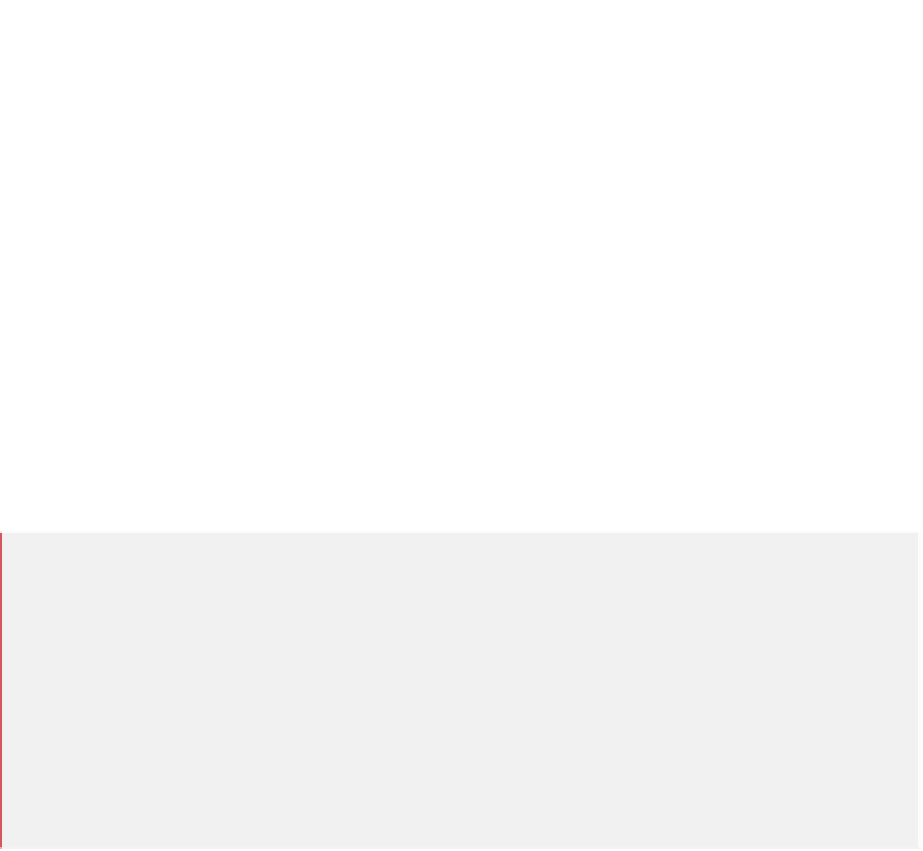













