Java Reference
In-Depth Information
• Class
OptionalDouble
's
getAsDouble
method returns the
double
in the object or throws a
NoSuchElementException
. To prevent this exception, you can call method
orElse
, which returns
the
OptionalDouble
's value if there is one, or the value you pass to
orElse
, otherwise.
•
IntStream
method
summaryStatistics
performs the
count
,
min
,
max
,
sum
and
average
opera-
tions in one pass of an
IntStream
's elements and returns the results as an
IntSummaryStatistics
object (package
java.util
).
Section 17.3.3 Terminal Operation
reduce
• You can define your own reductions for an
IntStream
by calling its
reduce
method. The first
argument is a value that helps you begin the reduction operation and the second argument is an
object that implements the
IntBinaryOperator
(p. 740) functional interface.
•Method
reduce
's first argument is formally called an identity value (p. 740)—a value that, when com-
bined with any stream element using the
IntBinaryOperator
produces that element's original value.
Section 17.3.4 Intermediate Operations: Filtering and Sorting
IntStream
Values
• You filter elements to produce a stream of intermediate results that match a predicate.
IntStream
method
filter
(p. 741) receives an object that implements the
IntPredicate
functional inter-
face (package
java.util.function
).
•
IntStream
method
sorted
(a lazy operation) orders the elements of the stream into ascending
order (by default). All prior intermediate operations in the stream pipeline must be complete so
that method
sorted
knows which elements to sort.
•Method
filter
a stateless intermediate operation—it does not require any information about
other elements in the stream in order to test whether the current element satisfies the predicate.
•Method
sorted
is a stateful intermediate operation that requires information about all of the oth-
er elements in the stream in order to sort them.
•Interface
IntPredicate
's
default
method
and
(p. 741) performs a logical AND operation with short-
circuit evaluation between the
IntPredicate
on which it's called and its
IntPredicate
argument.
• Interface
IntPredicate
's
default
method
negate
(p. 741) reverses the
boolean
value of the
Int-
Predicate
on which it's called.
• Interface
IntPredicate
default
method
or
(p. 741) performs a logical OR operation with short-
circuit evaluation between the
IntPredicate
on which it's called and its
IntPredicate
argument.
• You can use the interface
IntPredicate
default
methods to compose more complex conditions.
Section 17.3.5 Intermediate Operation: Mapping
• Mapping is an intermediate operation that transforms a stream's elements to new values and pro-
duces a stream containing the resulting (possibly different type) elements.
•
IntStream
method
map
(a stateless intermediate operation; p. 742) receives an object that imple-
ments the
IntUnaryOperator
functional interface (package
java.util.function
).
Section 17.3.6 Creating Streams of
int
s with
IntStream
Methods
range
and
rangeClosed
•
IntStream
methods
range
(p. 743) and
rangeClosed
each produce an ordered sequence of
int
values. Both methods take two
int
arguments representing the range of values. Method
range
produces a sequence of values from its first argument up to, but not including, its second argu-
ment. Method
rangeClosed
produces a sequence of values including both of its arguments.
Section 17.4
Stream<Integer>
Manipulations
• Class
Array
's
stream
method is used to create a
Stream
from an array of objects.
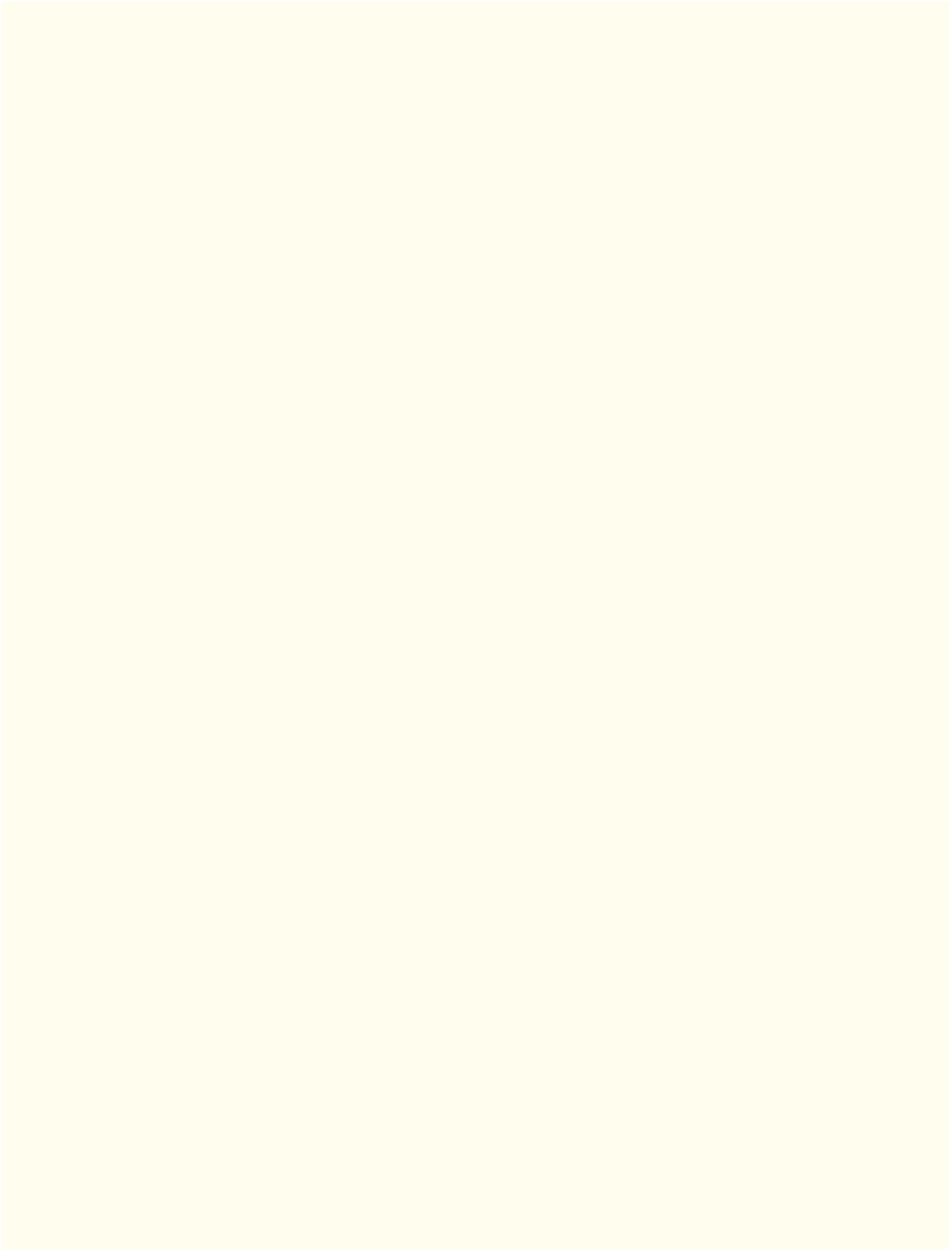