Java Reference
In-Depth Information
7
import
java.util.Scanner;
8
9
public class
WordTypeCount
10
{
11
public static void
main(String[] args)
12
{
13
// create HashMap to store String keys and Integer values
Map<String, Integer> myMap =
new
HashMap<>();
14
15
16
createMap(myMap);
// create map based on user input
17
displayMap(myMap);
// display map content
18
}
19
20
// create map from user input
21
private static void
createMap(Map<String, Integer> map)
22
{
23
Scanner scanner =
new
Scanner(System.in);
// create scanner
24
System.out.println(
"Enter a string:"
);
// prompt for user input
25
String input = scanner.nextLine();
26
27
// tokenize the input
28
String[] tokens = input.split(
" "
);
29
30
// processing input text
31
for
(String token : tokens)
32
{
33
String word = token.toLowerCase();
// get lowercase word
34
35
// if the map contains the word
36
if
(
map.containsKey(word)
)
// is word in map
37
{
38
int
count = map.get(word);
// get current count
map.put(word, count +
1
);
// increment count
39
40
}
41
else
42
map.put(word,
1
);
// add new word with a count of 1 to map
43
}
44
}
45
46
// display map content
47
private static void
displayMap(Map<String, Integer> map)
48
{
49
Set<String> keys = map.keySet();
// get keys
50
51
// sort keys
52
TreeSet<String> sortedKeys =
new
TreeSet<>(keys);
53
54
System.out.printf(
"%nMap contains:%nKey\t\tValue%n"
);
55
56
// generate output for each key in map
57
for
(String key : sortedKeys)
58
System.out.printf(
"%-10s%10s%n"
, key,
map.get(key)
);
59
Fig. 16.18
|
Program counts the number of occurrences of each word in a
String
. (Part 2 of 3.)
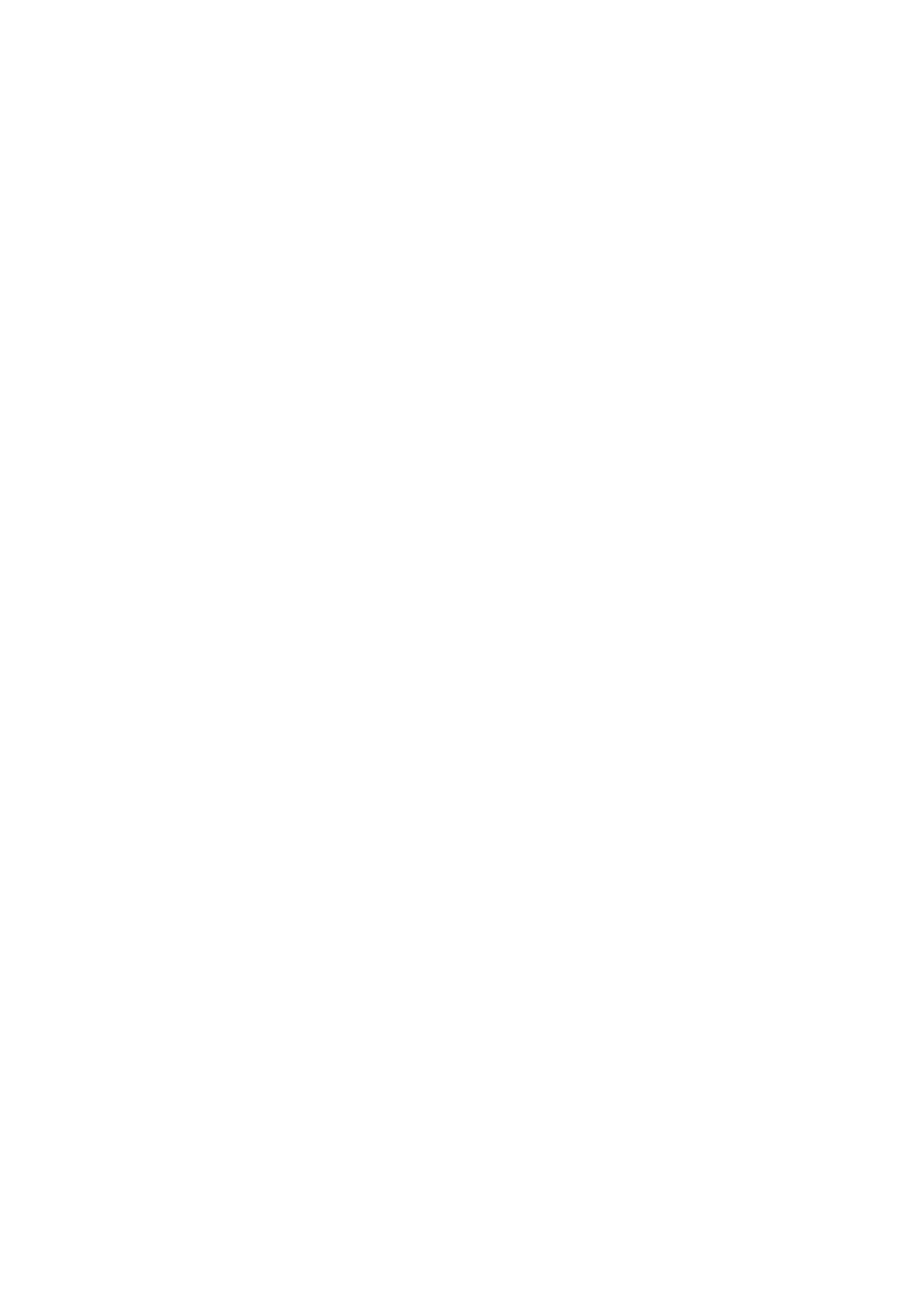



















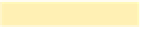

