Java Reference
In-Depth Information
14
15
int
minuteDifference = time1.getMinute() - time2.getMinute();
16
17
if
(minuteDifference !=
0
)
// then test the minute
18
return
minuteDifference;
19
20
int
secondDifference = time1.getSecond() - time2.getSecond();
21
return
secondDifference;
22
}
23
}
// end class TimeComparator
Fig. 16.8
|
Custom
Comparator
class that compares two
Time2
objects. (Part 2 of 2.)
Class
TimeComparator
implements interface
Comparator
, a generic type that takes
one type argument (in this case
Time2
). A class that implements
Comparator
must declare
a
compare
method that receives two arguments and returns a
negative
integer if the first
argument is
less
than
the second, 0 if the arguments are
equal
or a
positive
integer if the first
argument is
greater than
the second. Method
compare
(lines 7-22) performs comparisons
between
Time2
objects. Line 10 calculates the difference between the hours of the two
Time2
objects. If the hours are different (line 12), then we return this value. If this value is
positive
, then the first hour is greater than the second and the first time is greater than the
second. If this value is
negative
, then the first hour is less than the second and the first time
is less than the second. If this value is zero, the hours are the same and we must test the
minutes (and maybe the seconds) to determine which time is greater.
Figure 16.9 sorts a list using the custom
Comparator
class
TimeComparator
. Line 11
creates an
ArrayList
of
Time2
objects. Recall that both
ArrayList
and
List
are generic
types and accept a type argument that specifies the element type of the collection. Lines
13-17 create five
Time2
objects and add them to this list. Line 23 calls method
sort
,
passing it an object of our
TimeComparator
class (Fig. 16.8).
1
// Fig. 16.9: Sort3.java
2
// Collections method sort with a custom Comparator object.
3
import
java.util.List;
4
import
java.util.ArrayList;
5
import
java.util.Collections;
6
7
public class
Sort3
8
{
9
public static void
main(String[] args)
10
{
11
List<Time2> list =
new
ArrayList<>();
// create List
12
13
list.add(
new
Time2(
6
,
24
,
34
));
14
list.add(
new
Time2(
18
,
14
,
58
));
15
list.add(
new
Time2(
6
,
05
,
34
));
16
list.add(
new
Time2(
12
,
14
,
58
));
17
list.add(
new
Time2(
6
,
24
,
22
));
18
Fig. 16.9
|
Collections
method
sort
with a custom
Comparator
object. (Part 1 of 2.)
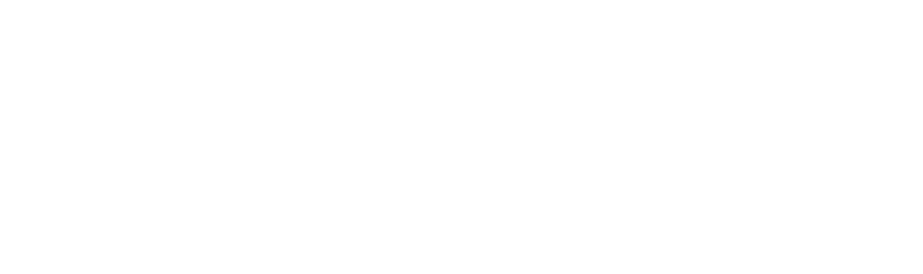
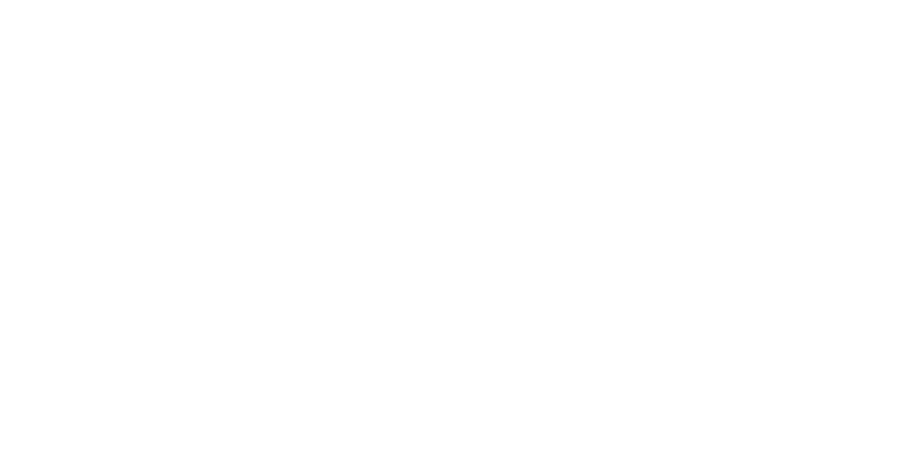



