Java Reference
In-Depth Information
15.1
Introduction
15.2
Files and Streams
15.3
Using NIO Classes and Interfaces to
Get File and Directory Information
15.4
Sequential-Access Text Files
15.4.1 Creating a Sequential-Access Text File
15.4.2 Reading Data from a Sequential-
Access Text File
15.4.3 Case Study: A Credit-Inquiry Program
15.4.4 Updating Sequential-Access Files
15.5
Object Serialization
15.5.1 Creating a Sequential-Access File
Using Object Serialization
15.5.2 Reading and Deserializing Data from a
Sequential-Access File
15.6
Opening Files with
JFileChooser
15.7
(Optional) Additional
java.io
Classes
15.7.1 Interfaces and Classes for Byte-Based
Input and Output
15.7.2 Interfaces and Classes for Character-
Based Input and Output
15.8
Wrap-Up
Summary | Self-Review Exercises | Answers to Self-Review Exercises | Exercises | Making a Difference
Data stored in variables and arrays is
temporary
—it's lost when a local variable goes out of
scope or when the program terminates. For long-term retention of data, even after the pro-
grams that create the data terminate, computers use
files
. You use files every day for tasks
such as writing a document or creating a spreadsheet. Computers store files on
secondary
storage devices
, including hard disks, flash drives, DVDs and more. Data maintained in
files is
persistent data
—it exists beyond the duration of program execution. In this chap-
ter, we explain how Java programs create, update and process files.
We begin with a discussion of Java's architecture for handling files programmatically.
Next we explain that data can be stored in
text files
and
binary files
—and we cover the dif-
ferences between them. We demonstrate retrieving information about files and directories
using classes
Paths
and
Files
and interfaces
Path
and
DirectoryStream
(all from package
java.nio.file
), then consider the mechanisms for writing data to and reading data from
files. We show how to create and manipulate sequential-access text files. Working with
text files allows you to quickly and easily start manipulating files. As you'll learn, however,
it's difficult to read data from text files back into object form. Fortunately, many object-
oriented languages (including Java) provide ways to write objects to and read objects from
files (known as
object serialization
and
deserialization
). To demonstrate this, we recreate
some of our sequential-access programs that used text files, this time by storing objects in
and retrieving objects from binary files.
Java views each file as a sequential
stream
of bytes
(Fig. 15.1).
1
Every operating system
provides a mechanism to determine the end of a file, such as an
end-of-file marker
or a
count of the total bytes in the file that's recorded in a system-maintained administrative
data structure. A Java program processing a stream of bytes simply receives an indication
from the operating system when it reaches the end of the stream—the program does
not
need to know how the underlying p
latform represents files or streams. In some cases, the
1.
Java's NIO APIs also include classes and interfaces that implement so-called channel-based architec-
ture for high-performance I/O. These topics are beyond the scope of this topic.
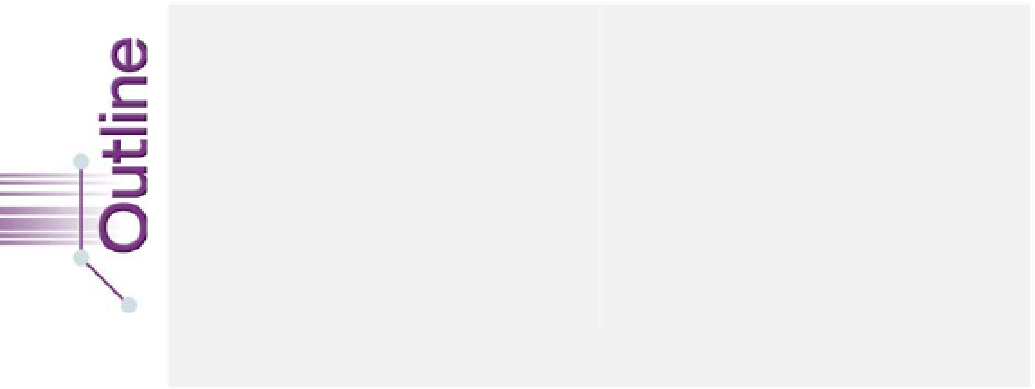


