Java Reference
In-Depth Information
1
// Fig. 13.5: ColorJPanel.java
2
// Changing drawing colors.
3
import
java.awt.Graphics;
4
5
import
java.awt.Color;
import
javax.swing.JPanel;
6
7
public
class
ColorJPanel
extends
JPanel
8
{
9
// draw rectangles and Strings in different colors
10
@Override
11
public
void
paintComponent(Graphics g)
12
{
13
super.paintComponent(g);
14
this
.setBackground(
Color.WHITE
);
15
16
// set new drawing color using integers
17
g.setColor(
new
Color(
255
,
0
,
0
));
g.fillRect(
15
,
25
,
100
,
20
);
g.getColor()
18
19
g.drawString(
"Current RGB: "
+
,
130
,
40
);
20
21
// set new drawing color using floats
22
g.setColor(
new
Color(
0.50f
,
0.75f
,
0.0f
));
23
g.fillRect(
15
,
50
,
100
,
20
);
24
g.drawString(
"Current RGB: "
+
g.getColor()
,
130
,
65
);
25
26
// set new drawing color using static Color objects
27
g.setColor(
Color.BLUE
);
28
g.fillRect(
15
,
75
,
100
,
20
);
29
g.drawString(
"Current RGB: "
+ g.getColor(),
130
,
90
);
30
31
// display individual RGB values
32
Color color =
Color.MAGENTA
;
g.setColor(color);
33
34
g.fillRect(
15
,
100
,
100
,
20
);
35
g.drawString(
"RGB values: "
+
color.getRed()
+
", "
+
36
color.getGreen()
+
", "
+
color.getBlue()
,
130
,
115
);
37
}
38
}
// end class ColorJPanel
Fig. 13.5
|
Changing drawing colors.
1
// Fig. 13.6: ShowColors.java
2
// Demonstrating Colors.
3
import
javax.swing.JFrame;
4
5
public
class
ShowColors
6
{
7
// execute application
8
public
static
void
main(String[] args)
9
{
Fig. 13.6
|
Demonstrating
Color
s. (Part 1 of 2.)








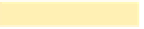


















