Java Reference
In-Depth Information
9
public static void
main(String[] args)
10
{
11
// create JFrame
12
JFrame application =
new
JFrame(
"A simple paint program"
);
13
14
PaintPanel paintPanel =
new
PaintPanel();
15
application.add(paintPanel,
BorderLayout.CENTER
);
16
17
// create a label and place it in SOUTH of BorderLayout
18
application.add(
new
JLabel(
"Drag the mouse to draw")
,
19
BorderLayout.SOUTH
);
20
21
application.setDefaultCloseOperation(
JFrame.EXIT_ON_CLOSE
);
22
application.setSize(
400
,
200
);
23
application.setVisible(
true
);
24
}
25
}
// end class Painter
Fig. 12.35
|
Testing
PaintPanel
. (Part 2 of 2.)
This section presents the
KeyListener
interface for handling
key events
. Key events are
generated when keys on the keyboard are pressed and released. A class that implements
KeyListener
must provide declarations for methods
keyPressed
,
keyReleased
and
key-
Typed
, each of which receives a
KeyEvent
as its argument. Class
KeyEvent
is a subclass of
InputEvent
. Method
keyPressed
is called in response to pressing any key. Method
key-
Typed
is called in response to pressing any key that is not an
action key
. (The action keys
are any arrow key,
Home
,
End
,
Page Up
,
Page Down
, any function key, etc.) Method
key-
Released
is called when the key is released after any
keyPressed
or
keyTyped
event.
The application of Figs. 12.36-12.37 demonstrates the
KeyListener
methods. Class
KeyDemoFrame
implements the
KeyListener
interface, so all three methods are declared in
the application. The constructor (Fig. 12.36, lines 17-28) registers the application to
handle its own key events by using method
addKeyListener
at line 27. Method
addKey-
Listener
is declared in class
Component
, so every subclass of
Component
can notify
Key-
Listener
objects of key events for that
Component
.
1
// Fig. 12.36: KeyDemoFrame.java
2
// Key event handling.
3
import
java.awt.Color;
Fig. 12.36
|
Key event handling. (Part 1 of 3.)
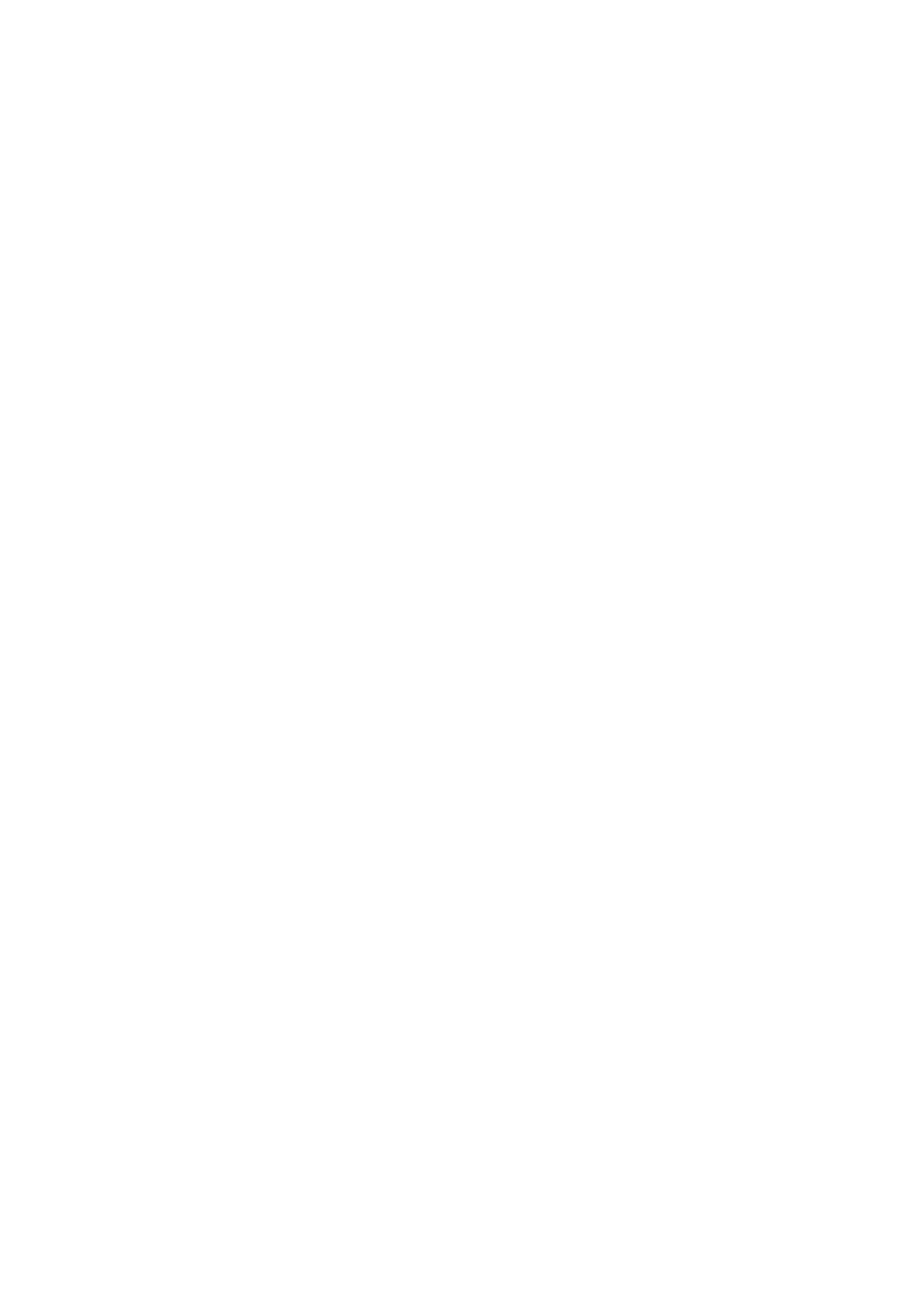

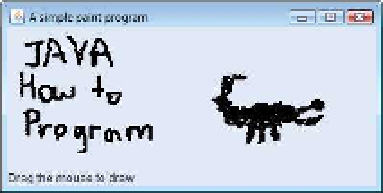




