Java Reference
In-Depth Information
11.9
This enables a program to catch related types of exceptions and process them in a uniform
manner. However, it's often useful to process the subclass types individually for more precise excep-
tion handling.
11.10
The
finally
block is the preferred means for releasing resources to prevent resource leaks.
11.11
First, control passes to the
finally
block if there is one. Then the exception will be pro-
cessed by a
catch
block (if one exists) associated with an enclosing
try
block (if one exists).
11.12
It rethrows the exception for processing by an exception handler of an enclosing
try
state-
ment, after the
finally
block of the current
try
statement executes.
11.13
The reference goes out of scope. If the referenced object becomes unreachable, the object
can be garbage collected.
Exercises
11.14
(Exceptional Conditions)
List the various exceptional conditions that have occurred in pro-
grams throughout this text so far. List as many additional exceptional conditions as you can. For
each of these, describe briefly how a program typically would handle the exception by using the ex-
ception-handling techniques discussed in this chapter. Typical exceptions include division by zero
and array index out of bounds.
11.15
(Exceptions and Constructor Failure)
Until this chapter, we've found dealing with errors
detected by constructors to be a bit awkward. Explain why exception handling is an effective means
for dealing with constructor failure.
11.16
(Catching Exceptions with Superclasses)
Use inheritance to create an exception superclass
(called
ExceptionA
) and exception subclasses
ExceptionB
and
ExceptionC
, where
ExceptionB
inher-
its from
ExceptionA
and
ExceptionC
inherits from
ExceptionB
. Write a program to demonstrate
that the
catch
block for type
ExceptionA
catches exceptions of types
ExceptionB
and
ExceptionC
.
11.17
(Catching Exceptions Using Class
Exception
)
Write a program that demonstrates how var-
ious exceptions are caught with
catch
(Exception exception)
This time, define classes
ExceptionA
(which inherits from class
Exception
) and
ExceptionB
(which
inherits from class
ExceptionA
). In your program, create
try
blocks that throw exceptions of types
ExceptionA
,
ExceptionB
,
NullPointerException
and
IOException
. All exceptions should be
caught with
catch
blocks specifying type
Exception
.
11.18
(Order of
catch
Blocks)
Write a program demonstrating that the order of
catch
blocks is
important. If you try to catch a superclass exception type before a subclass type, the compiler should
generate errors.
11.19
(Constructor Failure)
Write a program that shows a constructor passing information about
constructor failure to an exception handler. Define class
SomeClass
, which throws an
Exception
in
the constructor. Your program should try to create an object of type
SomeClass
and catch the ex-
ception that's thrown from the constructor.
11.20
(Rethrowing Exceptions)
Write a program that illustrates rethrowing an exception. Define
methods
someMethod
and
someMethod2
. Method
someMethod2
should initially throw an exception.
Method
someMethod
should call
someMethod2
, catch the exception and rethrow it. Call
someMethod
from method
main
, and catch the rethrown exception. Print the stack trace of this exception.
11.21
(Catching Exceptions Using Outer Scopes)
Write a program showing that a method with its
own
try
block does not have to catch every possible error generated within the
try
. Some exceptions
can slip through to, and be handled in, other scopes.
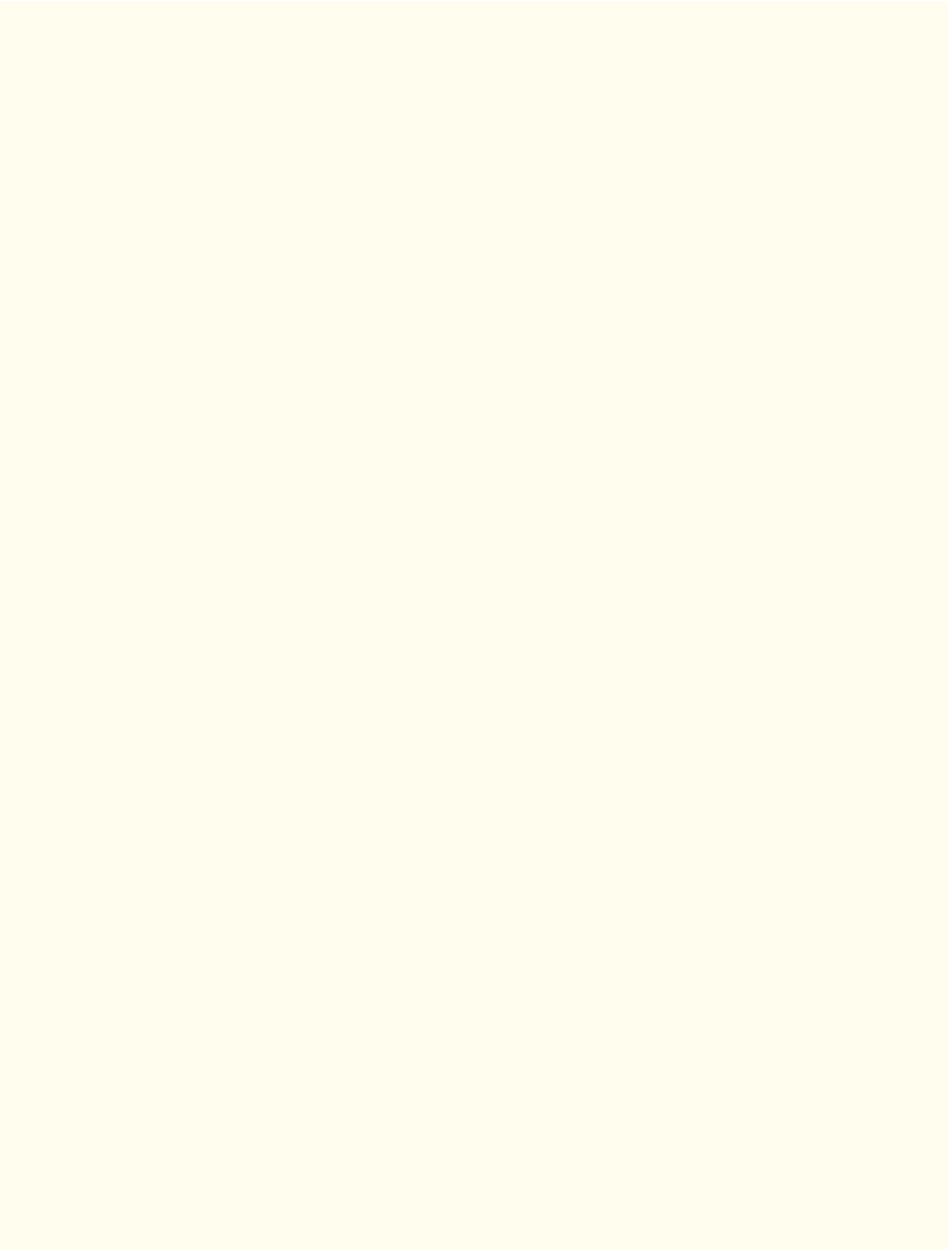